- epswing
- Nov 4, 2003
-
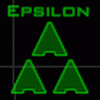
-
Soiled Meat
|
I think you want the Javascript thread...
|
#
?
Dec 1, 2009 18:46
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
?
May 8, 2024 23:11
|
|
- Mellomeh
- Jun 12, 2006
-
|
Quick question:
Say I had an ArrayList of 5 objects, and I wanted to loop through this ArrayList 5 times, starting from a different object each time. Is this possible?
So normally when I loop through once, it starts on the first object and works its way down to the fifth, I want to make it so that it then starts on the second and ends on the first, then starts on the third ends on the second etc.
Hope I explained this OK.
|
#
?
Dec 4, 2009 04:55
|
|
- HFX
- Nov 29, 2004
-
|
Mellomeh posted:
Quick question:
Say I had an ArrayList of 5 objects, and I wanted to loop through this ArrayList 5 times, starting from a different object each time. Is this possible?
So normally when I loop through once, it starts on the first object and works its way down to the fifth, I want to make it so that it then starts on the second and ends on the first, then starts on the third ends on the second etc.
Hope I explained this OK.
Since it sounds you are new to things, I broke this out into more lines then I probably would have written.
code:public void loop5TimesIncrementingStartEachTime(ArrayList arrayList) {
for (int loopCount = 0; loopCount < 5; loopCount++) {
arrayListLoop(loopCount, arrayList);
}
}
private void arrayListLoop(int startPosition, ArrayList arrayList) {
for (int index = 0; index < arrayList.size(); index++) {
int computedOffsetIndexValue = (index + startPosition) % arrayList.size();
Object value = arrayList.get(computedOffsetIndexValue);
doSomethingToItem(value);
}
}
private void doSomethingToItem(Object value) {
// Whatever you want to do.
}
|
#
?
Dec 4, 2009 05:21
|
|
- Mellomeh
- Jun 12, 2006
-
|
HFX posted:
Since it sounds you are new to things, I broke this out into more lines then I probably would have written.
code:public void loop5TimesIncrementingStartEachTime(ArrayList arrayList) {
for (int loopCount = 0; loopCount < 5; loopCount++) {
arrayListLoop(loopCount, arrayList);
}
}
private void arrayListLoop(int startPosition, ArrayList arrayList) {
for (int index = 0; index < arrayList.size(); index++) {
int computedOffsetIndexValue = (index + startPosition) % arrayList.size();
Object value = arrayList.get(computedOffsetIndexValue);
doSomethingToItem(value);
}
}
private void doSomethingToItem(Object value) {
// Whatever you want to do.
}
Thank you so much, I am fairly new to Java, so this is perfect.
|
#
?
Dec 4, 2009 05:31
|
|
- dis astranagant
- Dec 14, 2006
-
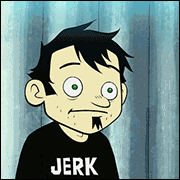
|
I'm having a bit of a brain fart at the moment and can't figure out the right way to convert:
code: for(Course c:courses)
for(Course d:courses){
if (c.conflicts(d)){
ts.conflict(c, d);
courses.remove(c);
courses.remove(d);
}
}
Into something that doesn't throw ConcurrentModificationException. courses is an ArrayList of Course objects, Course.conflicts() is a simple comparison function to make sure the two don't eat the same time slot, and ts.conflict() is just logs the event to a jTextArea for output. Any ideas?
|
#
?
Dec 4, 2009 06:07
|
|
- HFX
- Nov 29, 2004
-
|
dis astranagant posted:
I'm having a bit of a brain fart at the moment and can't figure out the right way to convert:
code: for(Course c:courses)
for(Course d:courses){
if (c.conflicts(d)){
ts.conflict(c, d);
courses.remove(c);
courses.remove(d);
}
}
Into something that doesn't throw ConcurrentModificationException. courses is an ArrayList of Course objects, Course.conflicts() is a simple comparison function to make sure the two don't eat the same time slot, and ts.conflict() is just logs the event to a jTextArea for output. Any ideas?
You need to think about your code before you ever think about calling this. For one, c will conflict with itself from d. Secondly, the second iterator is invalid once you remove the item. You would need to build a list and then remove the conflicts after. You should also preform removals using the iterators method.
Personally, I would overload equals and use a set to keep the conflicts from happening in the first place.
Remember kids, the foreach for loop is just syntactic sugar for getting an iterator and using a while loop.
HFX fucked around with this message at 08:09 on Dec 4, 2009
|
#
?
Dec 4, 2009 07:58
|
|
- Volguus
- Mar 3, 2009
-
|
dis astranagant posted:
I'm having a bit of a brain fart at the moment and can't figure out the right way to convert:
code: for(Course c:courses)
for(Course d:courses){
if (c.conflicts(d)){
ts.conflict(c, d);
courses.remove(c);
courses.remove(d);
}
}
Into something that doesn't throw ConcurrentModificationException. courses is an ArrayList of Course objects, Course.conflicts() is a simple comparison function to make sure the two don't eat the same time slot, and ts.conflict() is just logs the event to a jTextArea for output. Any ideas?
I do not know for sure if what you're doing there is correct or not since I don't have the bigger picture, but when I usually want to remove elements from a list while traversing through it i do something like:
code:for(int i=list.size()-1;i>=0;i--)
{
if(somecondition)
{
list.remove(i);
}
}
Using an iterator and calling its remove method will work too, of course.
|
#
?
Dec 4, 2009 15:53
|
|
- Mustach
- Mar 2, 2003
-
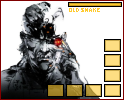
In this long line, there's been some real strange genes. You've got 'em all, with some extras thrown in.
|
java.util.Arrays.copyOf()
java.util.Arrays.copyOfRange()
java.lang.System.arrayCopy()
The first two create the copy, the third takes the destination as a parameter
|
#
?
Dec 4, 2009 21:38
|
|
- Rocko Bonaparte
- Mar 12, 2002
-
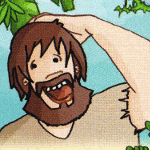
Every day is Friday!
|
I have a memory leak in a large Java program. I got an hprof dump and used an Eclipse analyzer to try to make sense of it. I am finding a JDBC ResultSet object is dangling around. I don't know if that's the single problem, but it shouldn't be there, and I want to figure out how to sever it.
My program is making a few huge queries to a database using a singleton to facilitate all communication to the database. From these queries I get ResultSet objects to gobble up everything into the data structure my program needs. The singleton is a warning sign right there. All ResultSets I instantiate are local to the methods in which they are called. Before leaving the method, I will close the ResultSet, and try to close it when an Exception occurs. That's the same for the database connection. Generally each method looks something like:
code:public xyz method(abc) throws Exception {
DBConnection connection = null; // Dunno if that's the right name for a JDBC connection
ResultSet results = null;
try {
connection = ... set up connection ...
results = ... results from a query ...
... do stuff with results ...
results.close();
connection.close();
} catch(Exception e) {
if(results != null) {
results.close();
}
if(connection != null) {
connection.close();
}
throw e;
}
return whatever;
}
Despite this it looks like a ResultSet will persist into the second phase of the application where I no longer call the database. Nothing from the singleton return a ResultSet to the caller.
All I can think of doing now is after closing the results and connection that I set the reference to null. Is there anything out I should watch out for?
|
#
?
Dec 6, 2009 22:42
|
|
- Fly
- Nov 3, 2002
-
 moral compass
|
Use finally to close resources.
code:Connection foo = null;
try {
foo = getConnection();
// stuff
}
catch () {
// stuff
}
finally {
if (foo != null)
foo.close();
}
Be sure to close your Statements, too. edit: Also be sure your finally is not throwing exceptions when you are closing multiple things, but if you close the Connection, you're closing the ResultSet.
code:finally {
try {
if (rs != null)
rs.close();
} catch (Exception ex) { // waaah }
finally {
if (foo != null)
foo.close();
}
}
You can write a safeClose(ResultSet rs) that catches the exception if you have to repeat this pattern a lot.
Fly fucked around with this message at 07:01 on Dec 7, 2009
|
#
?
Dec 7, 2009 06:56
|
|
- zootm
- Aug 8, 2006
-
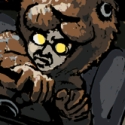 We used to be better friends.
|
Fly posted:
You can write a safeClose(ResultSet rs) that catches the exception if you have to repeat this pattern a lot.
One of the Apache Commons packages provides IOUtils.closeQuietly for this.
zootm fucked around with this message at 11:11 on Dec 7, 2009
|
#
?
Dec 7, 2009 09:27
|
|
- Rocko Bonaparte
- Mar 12, 2002
-
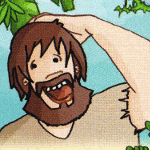
Every day is Friday!
|
I ended up writing a helper that not only closed the connections but set the pointers to null. That nuked the stray ResultSet that was laying around. I ended up having some danglers of my own which were probably the more fundamental problem. I figured I'd share.
If you have a list of jobs to do of some kind, it's probably better for the resources you have to dequeue them and dismiss the old references rather than using, say, a for loop with an index. The completed jobs hang around afterwards because they're still in the data structure. In my case, the jobs started as about 4kB of memory and ballooned to about 120MB after they were finished. By removing the references the GC was able to see them as unused anymore and reclaim them.
|
#
?
Dec 7, 2009 20:23
|
|
- trex eaterofcadrs
- Jun 17, 2005
-
My lack of understanding is only exceeded by my lack of concern.
|
Rocko Bonaparte posted:
I ended up writing a helper that not only closed the connections but set the pointers to null. That nuked the stray ResultSet that was laying around. I ended up having some danglers of my own which were probably the more fundamental problem. I figured I'd share.
If you have a list of jobs to do of some kind, it's probably better for the resources you have to dequeue them and dismiss the old references rather than using, say, a for loop with an index. The completed jobs hang around afterwards because they're still in the data structure. In my case, the jobs started as about 4kB of memory and ballooned to about 120MB after they were finished. By removing the references the GC was able to see them as unused anymore and reclaim them.
WeakReference is your friend.
|
#
?
Dec 7, 2009 20:37
|
|
- nonathlon
- Jul 9, 2004
-
And yet, somehow, now it's my fault ...
|
Two possibly naive questions from a Java neophyte:
1. I've gotten interested in dynamic languages running on the JVM (for development speed, quick scripts, data analysis etc. as well as for learning another language). Coming from Python, Jython is the obvious choice but it seems to be a 2nd class citizen - just a bit messy, some standard libraries not complete, a bit slow, doesn't seem to have a huge development effort behind it. JRuby? Also a bit slow, and I'm a bit concerned about Ruby oddities. So my eye has been drawn to Groovy and Scala. Scala in particular looks cool, and promises to be fast for a dynamic language. Any opinions or things I should look out for? What are the distinguishing points or USPs between Groovy and Scala?
2. What numerics, stats and graphing libraries would people recommend within Java? In Python, there's just a few obvious leaders that everyone uses (Numpy and matplotlib), but I haven't been able to sort the best options from the wide variety available for Java.
|
#
?
Dec 7, 2009 20:43
|
|
- Fly
- Nov 3, 2002
-
 moral compass
|
That could be a bad idea though. If the jobs have not been completed, but they are only weakly reachable, the garbage collector could get rid of them before they are executed. In the case of a work queue, I suggest a producer/consumer model for most situations.
|
#
?
Dec 7, 2009 20:44
|
|
- trex eaterofcadrs
- Jun 17, 2005
-
My lack of understanding is only exceeded by my lack of concern.
|
Fly posted:
That could be a bad idea though. If the jobs have not been completed, but they are only weakly reachable, the garbage collector could get rid of them before they are executed. In the case of a work queue, I suggest a producer/consumer model for most situations.
Yeah I thought about that once I posted. Oops.
If they're non-critical you could use a SoftReference instead, I suppose.
|
#
?
Dec 7, 2009 20:50
|
|
- Flamadiddle
- May 9, 2004
-
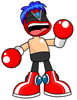
|
Okay, I have no idea what's going on but I'm all of a sudden having real difficulty with packages and classpaths under Windows 7.
I've just tested the following:
code:package mypack;
public class a{
public a(){
System.out.println("found me");
}
}
and class b:
code:package mypack;
public class b{
public static void main(String[] args){
a mya = new a();
}
}
I'd expect b to run, find a in its package and construct it, but I get cannot find symbol errors. It works if b is outside the package and imports a, but not this way. This is really bugging me and I can't figure it out. Any suggestions?
Edit: It seems to be a problem with the way I was compiling. if I specify the classpath as .. when I compile within the mypack folder it compiles fine. I must have the classpath set differently on my other pc, I guess.
Flamadiddle fucked around with this message at 02:06 on Dec 8, 2009
|
#
?
Dec 8, 2009 01:36
|
|
- ynef
- Jun 12, 2002
-
|
Flamadiddle posted:
Okay, I have no idea what's going on but I'm all of a sudden having real difficulty with packages and classpaths under Windows 7.
I've just tested the following:
code:package mypack;
public class a{
public a(){
System.out.println("found me");
}
}
and class b:
code:package mypack;
public class b{
public static void main(String[] args){
a mya = new a();
}
}
I'd expect b to run, find a in its package and construct it, but I get cannot find symbol errors. It works if b is outside the package and imports a, but not this way. This is really bugging me and I can't figure it out. Any suggestions?
Edit: It seems to be a problem with the way I was compiling. if I specify the classpath as .. when I compile within the mypack folder it compiles fine. I must have the classpath set differently on my other pc, I guess.
Nope, that's what is supposed to happen: the class path should point to a location where the subdirectories are the same as the package names of the classes that you want to use.
|
#
?
Dec 9, 2009 19:31
|
|
- Flamadiddle
- May 9, 2004
-
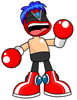
|
Yeah, I figured that much out, thanks. Can't believe it took me so long.
|
#
?
Dec 10, 2009 00:38
|
|
- PT6A
- Jan 5, 2006
-
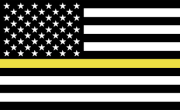
Public school teachers are callous dictators who won't lift a finger to stop children from peeing in my plane
|
ynef posted:
Nope, that's what is supposed to happen: the class path should point to a location where the subdirectories are the same as the package names of the classes that you want to use.
Speaking of which, is there any way to circumvent this requirement? In my recent software design project (a simple card game), one of the bonus features we implemented was to have AI classes loaded dynamically from .class files. We did this, but it was a pain in the rear end to have to have them in a properly named subdirectory. I suppose the proper way to do this is insist they be properly packaged in a separate JAR?
|
#
?
Dec 10, 2009 00:59
|
|
- rjmccall
- Sep 7, 2007
-
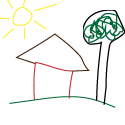
no worries friend
-
Fun Shoe
|
Use a custom ClassLoader subclass; just feed it a bucket of bytes and (if appropriate) the fully-qualified class you expected to find, and it'll give you a class back.
|
#
?
Dec 10, 2009 02:38
|
|
- StickFigs
- Sep 5, 2004
-
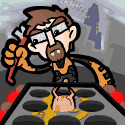
"It's time to choose."
|
I need to count the number of comparisons and swaps(aka exchanges) that occur in this code when sorting an int array:
code:/** Implements the insertion sort algorithm.
* @author Koffman and Wolfgang
* */
public class InsertionSort {
/** Sort the table using insertion sort algorithm.
pre: table contains Comparable objects.
post: table is sorted.
@param table The array to be sorted
*/
public static < T
extends Comparable < T >> void sort(T[] table) {
for (int nextPos = 1; nextPos < table.length; nextPos++) {
// Invariant: table[0 . . . nextPos - 1] is sorted.
// Insert element at position nextPos
// in the sorted subarray.
insert(table, nextPos);
} // End for.
} // End sort.
/** Insert the element at nextPos where it belongs
in the array.
pre: table[0 . . . nextPos - 1] is sorted.
post: table[0 . . . nextPos] is sorted.
@param table The array being sorted
@param nextPos The position of the element to insert
*/
private static < T
extends Comparable < T >> void insert(T[] table,
int nextPos) {
T nextVal = table[nextPos]; // Element to insert.
while (nextPos > 0
&& nextVal.compareTo(table[nextPos - 1]) < 0) {
table[nextPos] = table[nextPos - 1]; // Shift down.
nextPos--; // Check next smaller element.
}
// Insert nextVal at nextPos.
table[nextPos] = nextVal;
}
}
Where the hell do I put the numSwaps++ and numComparison++('s)?
|
#
?
Dec 10, 2009 05:11
|
|
- Brain Candy
- May 18, 2006
-
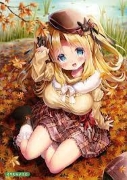
|
StickFigs posted:
I need to count the number of comparisons and swaps(aka exchanges) that occur in this code when sorting an int array:
*code*
Where the hell do I put the numSwaps++
Does insertion sort work by swapping?
Homework dude posted:
and numComparison++('s)?
Look for compareTo.
|
#
?
Dec 10, 2009 05:41
|
|
- StickFigs
- Sep 5, 2004
-
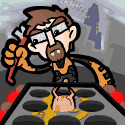
"It's time to choose."
|
Brain Candy posted:
Does insertion sort work by swapping?
Well it's just one array so somethings getting swapped.
|
#
?
Dec 10, 2009 06:29
|
|
- Brain Candy
- May 18, 2006
-
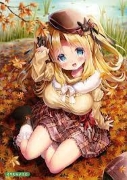
|
reordered!=swapped
Imagine four balls on the edge of a cliff... insertion sort is the same way.
|
#
?
Dec 10, 2009 06:42
|
|
- StickFigs
- Sep 5, 2004
-
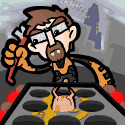
"It's time to choose."
|
Whatever I don't care what its called I just need to count them.
|
#
?
Dec 10, 2009 06:45
|
|
- epswing
- Nov 4, 2003
-
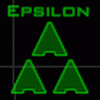
-
Soiled Meat
|
I'd probably start by writing insertion sort myself from scratch, using an int[] as input, just to understand what's going on. You can use the algorithm described in http://en.wikipedia.org/wiki/Insertion_sort nearly line-for-line.
code:import java.util.Arrays;
public class Blah {
public static void main(String[] args) {
int[] array = { 2, 6, 5, 1, 3, 2, 5, 2, 3 };
System.out.println(Arrays.toString(array)); // jumbled
sort(array);
System.out.println(Arrays.toString(array)); // should be sorted
}
public static void sort(int[] array) {
// fill this in
}
}
If you can write that, you'll see that the code you posted above just parts out the inner loop into another function, and it should then be easy to figure out where swaps and insertions occur.
|
#
?
Dec 10, 2009 06:52
|
|
- StickFigs
- Sep 5, 2004
-
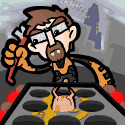
"It's time to choose."
|
According to this I should get 26 "Exchanges" and 32 "Comparisons" with this array:
int [] arr1 = {4,8,3,7,6,1,2,5,0,9}
With my code:
code:public class InsertionSort implements Sort {
private int numComparisons = 0;
private int numSwaps = 0;
public int getNumComparisons()
{
return numComparisons;
}
public int getNumSwaps()
{
return numSwaps;
}
public void resetStats()
{
numComparisons = 0;
numSwaps = 0;
System.out.println("-STATS RESET-");
}
public void sortHW8(int[] arr)
{
///---START INPUT---
System.out.print("IN: {");
for (int ent: arr)
{
System.out.print(ent);
if (ent == arr[arr.length-1])
{
}
else
{
System.out.print(", ");
}
}
System.out.print("}");
///---END INPUT---
for (int nextPos = 1; nextPos < arr.length; nextPos++)
{
insert(arr, nextPos);
}
///---START OUTPUT---
System.out.println();
System.out.print("OUT: {");
for (int ent: arr)
{
System.out.print(ent);
if (ent == arr[arr.length-1])
{
}
else
{
System.out.print(", ");
}
}
System.out.println("}");
///---END OUTPUT---
}
public void insert(int[] arr, int nextPos)
{
int nextVal = arr[nextPos]; // Element to insert.
while (nextPos > 0 && nextVal < arr[nextPos - 1])
{
numComparisons++;
arr[nextPos] = arr[nextPos - 1]; // Shift down.
numSwaps++;
nextPos--; // Check next smaller element.
}
// Insert nextVal at nextPos.
arr[nextPos] = nextVal;
}
}
I get 26 for both numbers even though I put the numComparisons++ right next to the only code where I can see array entries being compared. Where did I gently caress up?
|
#
?
Dec 10, 2009 07:07
|
|
- zootm
- Aug 8, 2006
-
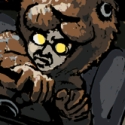 We used to be better friends.
|
PT6A posted:
Speaking of which, is there any way to circumvent this requirement? In my recent software design project (a simple card game), one of the bonus features we implemented was to have AI classes loaded dynamically from .class files. We did this, but it was a pain in the rear end to have to have them in a properly named subdirectory. I suppose the proper way to do this is insist they be properly packaged in a separate JAR?
While rjmccall's suggestion will work, I'd urge you to consider the JAR route; they're easy to build and prevent you needing to insist on your dynamically loaded modules being a single class, which is very restrictive.
|
#
?
Dec 10, 2009 09:28
|
|
- tef
- May 30, 2004
-
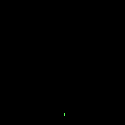
-> some l-system crap ->
|
Any recommended json libraries or such?
|
#
?
Dec 10, 2009 12:26
|
|
- Mustach
- Mar 2, 2003
-
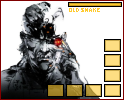
In this long line, there's been some real strange genes. You've got 'em all, with some extras thrown in.
|
tef posted:
Any recommended json libraries or such?
I just use org.json's stuff. It's not my favorite API, but it isn't painful, either. But maybe you're looking for something more sophisticated?
|
#
?
Dec 10, 2009 12:51
|
|
- geeves
- Sep 16, 2004
-
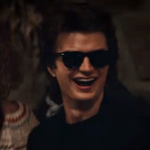
|
tef posted:
Any recommended json libraries or such?
I've been looking at this as well and currently have been comparing json.org/java vs json-lib.sourceforge.net (based on json.org but probably a bit more developed, according to them) and Jackson http://jackson.codehaus.org/
There is also one in Sling, but it's a bit limited and geared toward working with a JCR.
|
#
?
Dec 10, 2009 16:42
|
|
- geeves
- Sep 16, 2004
-
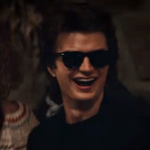
|
I have a question about performance and loops. Right now it works, but I'm looking for efficiency.
I guess this is more general programming than Java, but here it goes.
I am getting a list of tags, looping through them and then looping through the list of pages each of them return. I'm working with a Java Content Repository. So I have a loop within a loop. I've never thought this was ideal.
I'm still a somewhat Java beginner when it comes to certain concepts, but I hate nested IF statements, especially when checking for NULL. Should I leave that to the catch / exception handling? If something is null I don't want it to kill the whole loop, just ignore it (and log it, of course).
Should the second loop be another method or does that not matter? Is it better to have a nested try/catch/ finally? Should these be in a separate method? Is it personal preference? Or just something I'm stuck with?
Besides working, I like to go for readability and I know I'm not perfect in that area, but I do try my best.
code:Iterator<Entry<String, Integer>> it = tagMapFromContent.entrySet().iterator();
HashMap<String, Integer> contentWeight = new HashMap<String, Integer>();
try {
tagLoop: while (it.hasNext()) {
Entry<String, Integer> e = it.next();
Tag tag = getTagFromPath(e.getKey(), resourceResolver);
if (null != tag) {
pageLoop: while (res.hasNext()) {
Integer weight = tagMapFromContent.get(tag.getPath());
Resource r = res.next();
// Node n = r.adaptTo(Node.class);
String path = r.adaptTo(Node.class).getParent().getPath();
Page page = resourceResolver.getResource(path).adaptTo(Page.class);
if (null != page) {
//more logic
}
}
}
} catch(Exception e) {
//handle exception
}
|
#
?
Dec 15, 2009 05:59
|
|
- HFX
- Nov 29, 2004
-
|
geeves posted:
I have a question about performance and loops. Right now it works, but I'm looking for efficiency.
I guess this is more general programming than Java, but here it goes.
I am getting a list of tags, looping through them and then looping through the list of pages each of them return. I'm working with a Java Content Repository. So I have a loop within a loop. I've never thought this was ideal.
I'm still a somewhat Java beginner when it comes to certain concepts, but I hate nested IF statements, especially when checking for NULL. Should I leave that to the catch / exception handling? If something is null I don't want it to kill the whole loop, just ignore it (and log it, of course).
Should the second loop be another method or does that not matter? Is it better to have a nested try/catch/ finally? Should these be in a separate method? Is it personal preference? Or just something I'm stuck with?
Besides working, I like to go for readability and I know I'm not perfect in that area, but I do try my best.
code:Iterator<Entry<String, Integer>> it = tagMapFromContent.entrySet().iterator();
HashMap<String, Integer> contentWeight = new HashMap<String, Integer>();
try {
tagLoop: while (it.hasNext()) {
Entry<String, Integer> e = it.next();
Tag tag = getTagFromPath(e.getKey(), resourceResolver);
if (null != tag) {
pageLoop: while (res.hasNext()) {
Integer weight = tagMapFromContent.get(tag.getPath());
Resource r = res.next();
// Node n = r.adaptTo(Node.class);
String path = r.adaptTo(Node.class).getParent().getPath();
Page page = resourceResolver.getResource(path).adaptTo(Page.class);
if (null != page) {
//more logic
}
}
}
} catch(Exception e) {
//handle exception
}
I'm having a bit of trouble figuring out the bigger picture of what you are doing (which could help me recommend something better for you).
So I'll talk you a bit about what I do get.
geeves posted:
I have a question about performance and loops. Right now it works, but I'm looking for efficiency.
First efficiency is something to be careful about. How often is this running as part of your whole program? Are you spending an inordinate amount of time in it and why?
Now for your code:
The loops themselves would probably be better labeled using a comment or even better your method has a descriptive name minimizing the need for a comment. The inner loop is a good target for a private method. Java doesn't support private methods for a given method, so you will have to put it in the class itself. The outer loop could be also, but I can't see your whole method.
Do not worry about checking for nulls. This is actually how you want to write your code if you expect nulls. Avoid throwing exceptions except in exceptional circumstances.
With more knowledge about what you are trying to achieve, I possibly could recommend a better way of solving it, however, there is nothing super wrong about code.
|
#
?
Dec 15, 2009 06:24
|
|
- geeves
- Sep 16, 2004
-
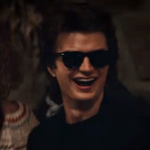
|
HFX posted:
I'm having a bit of trouble figuring out the bigger picture of what you are doing (which could help me recommend something better for you).
A simple weighting system for recommending an item for a user.
I have, for example, 7 tags on an Item. Each of those tags maybe used on 40 or 50 other Items. If an item already exists in the HashMap, then its weight (the Integer) is added. If it's a certain "mega" Item, its weight is +2 instead of +1. That's a simple if/else in the if (null != page)
quote:
So I'll talk you a bit about what I do get.
First efficiency is something to be careful about. How often is this running as part of your whole program? Are you spending an inordinate amount of time in it and why?
It runs only when a user saves an item to their cart. I don't know how often this will run. We know very little of the behavior of our visitors aside from what basic HTTP stats will tell us. Our site however gets upwards of 600-700k unique visits per month and 4-5 million page views per month. How many people will register and save content - I don't know. Nobody does and yes, that is the problem.
This is an entirely new feature and we're going from a completely static HTML site (managed with a "baked" CMS) to a JCR-based content repository. Basically I'm thinking worst case scenario.
quote:
Now for your code:
The loops themselves would probably be better labeled using a comment or even better your method has a descriptive name minimizing the need for a comment. The inner loop is a good target for a private method. Java doesn't support private methods for a given method, so you will have to put it in the class itself. The outer loop could be also, but I can't see your whole method.
That is the whole method, minus an if/else statement in the inner loop. I used to write a lot of loops for some hosed up business logic that needed to break the outer loop, so it's an old habit to label them in that way.
quote:
Do not worry about checking for nulls. This is actually how you want to write your code if you expect nulls. Avoid throwing exceptions except in exceptional circumstances.
That's been the problem with development, we have so much content and items that it's several gigs to import onto our local machines. That and there were several iterations of importing (to find the best structure in the JCR) so it was just easier to check for null or else it would throw a NullPointer and kill action.
quote:
With more knowledge about what you are trying to achieve, I possibly could recommend a better way of solving it, however, there is nothing super wrong about code.
|
#
?
Dec 15, 2009 17:03
|
|
- Lysidas
- Jul 26, 2002
-
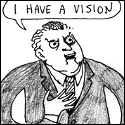
John Diefenbaker is a madman who thinks he's John Diefenbaker.
-
Pillbug
|
I'm under the impression that in Java*, exception handling is slow compared to conditional statements and that it is not intended to be part of the normal flow of program execution.
In general, you should only throw and expect to catch an exception if something ... exceptional happens. If having a null value in your collection is normal and common, don't write code as if it won't happen and catch the NullPointerException.
*Silly Python with its StopIteration exceptions. I kid; I love Python and think that this is a perfectly reasonable way to implement something like this, especially if exception handling is designed to be fast enough for this to be feasible.
|
#
?
Dec 16, 2009 07:57
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
?
May 8, 2024 23:11
|
|
- Fly
- Nov 3, 2002
-
 moral compass
|
Lysidas posted:
I'm under the impression that in Java*, exception handling is slow compared to conditional statements and that it is not intended to be part of the normal flow of program execution.
In general, you should only throw and expect to catch an exception if something ... exceptional happens. If having a null value in your collection is normal and common, don't write code as if it won't happen and catch the NullPointerException.
*Silly Python with its StopIteration exceptions. I kid; I love Python and think that this is a perfectly reasonable way to implement something like this, especially if exception handling is designed to be fast enough for this to be feasible.
Correct. The last time I tested this, throwing an exception was at least two orders of magnitude slower than using a conditional. Sometimes a 100x slowdown does not matter. However, the slow part is generally creating a new Throwable since the JVM has to figure out the stack, so if you throw an existing Throwable, or a singleton instance of one, it won't be so bad, and you could do that very easily when the exception does not originate from an exceptional condition. It's still not idiomatic Java style, so I would avoid it.
|
#
?
Dec 16, 2009 20:28
|
|