- boner confessor
- Apr 25, 2013
-
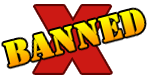
by R. Guyovich
|
i'm trying to learn python and i'm still very babbyish. i did something like this
code:## vehicle class
class Vehicle(object):
def name(self):
print "this is a vehicle!" #test
pass
## car subclass
class Car(Vehicle):
def __init__(self, name):
self.name = name
pass
## model sub subclass
class Model(Car):
def __init__(self, passenger_cap):
self.passenger_cap = passenger_cap
passenger_cap = int(4)
pass
what i'm trying to do is create different objects and write them to an array. i differentiate between Car and Truck or whatever so I can randomly generate different sets of names. that works fine
when i print out the records in my array, everything in the model subclass returns the output "<bound method model.name of <__main__.model at blah blah memory address" i get that it's returning the metadata about the attribute i want instead of the value of that attribute because it's not inheriting correctly, but i'm having a hard time figuring out exactly how i'm making this mistake
also please feel free to point out any other mistakes and bad conventions i'm doing here, i just started learning how to code
|
#
¿
Dec 16, 2014 06:36
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 19, 2024 22:39
|
|
- boner confessor
- Apr 25, 2013
-
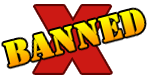
by R. Guyovich
|
i'm trying to make a palindrome tester, what am i doing wrong here?
code:def palindromeCheck(rawString):
#convert input to lowercase
lowerString = rawString.lower()
#clean out any punctuation
cleanString = lowerString.translate(None, '! .,')
#find half the length of the result
searchLength = len(cleanString) / 2
#compare char to char, starting from the middle and working outwards, ignoring the middle char of odd numbered strings
while searchLength > 0:
if cleanString[searchLength] == cleanString[-searchLength]:
searchLength -= 1
else:
return False
return True
rawString = raw_input("Give me a Palindrome: ")
isPalindrome = palindromeCheck(rawString)
if isPalindrome == True:
print "This is a Palindrome!"
else:
print "Sorry, this is not a Palindrome."
the problem is i can't get it to iterate through the if, i had assumed "while searchLength is still greater than 0 keep doing the if"
i managed to get it to work much more cleanly with a for
code:isPalindrome = True
#compare char to char, starting from the outside and working in, ignoring the middle char of odd numbered strings
for n in range (0, int(len(cleanString) / 2)):
if cleanString[n] != cleanString[-n-1]:
isPalindrome = False
break
return isPalindrome
but where did i go wrong with the first attempt?
|
#
¿
Oct 13, 2015 08:08
|
|
- boner confessor
- Apr 25, 2013
-
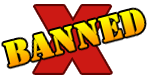
by R. Guyovich
|
The "return True" is over indented and inside the while loop, so your code will either return True or False on the first iteration (see Hammerite's analysis). You should be good if you remove an indent and have "return True" at the same level as the while loop.
i thought i tried that, huh. so if i do that, then 'while the counter is whatever if comparison is still valid deincrement searchLength and try again' is the expected behavior?
e: haha, that was the correct placement of the return in my previous test, but i forgot to test for str[index] == str[index-1]], and one of my test words was 'banana', which is a valid offset palindrome under that logic...
boner confessor fucked around with this message at 15:57 on Oct 13, 2015
|
#
¿
Oct 13, 2015 15:47
|
|
- boner confessor
- Apr 25, 2013
-
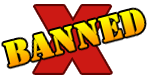
by R. Guyovich
|
both of these just flip the string and test that against the input, right? i figured that was more efficient but i wanted to do a step by step comparison
Python code:def is_palindrome(x):
return ''.join(reversed(x)) == x
return is a built in function that produces a bool
'' creates a new empty string?
.join fills in the new string with the reversed input x
== x tests to see if this new string is the same as the input string
Python code:def is_palindrome(x):
return x == x[::-1]
and this does the same thing, except using string slicing to start from the end and work backwards at an interval of 1 char per char, effectively reversing the string?
thanks all
|
#
¿
Oct 15, 2015 06:28
|
|