- lmao zebong
- Nov 25, 2006
-
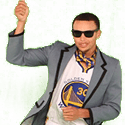
NBA All-Injury First Team
|
So, I'm stuck on this compiler error, and google is giving me no help.
I'm still pretty new to C++, only about 4 weeks into my first programming class. This is a practice problem out of the back of the book, and I'm having a hell of a time figuring out what the hell the compiler wants me to do!
here is my code:
code:#include <iostream>
using namespace std;
int main( )
{
double wages(16.78), overtime(25.17), sst(.05), fet(.14);
int union(10);
int numberDependents(0), hoursWorked(0);
cout.setf(ios::fixed);
cout.setf(ios::showpoint);
cout.precision(2);
cout << "Please enter the total hours worked this week:";
cin >> hoursWorked;
cout << "Please enter the number of dependents you have:";
cin >> numberDependents;
double weeklyPay, socialSecurity, federalIncome, netPay, netpayD;
int dep3(35);
weeklyPay = wages * hoursWorked;
socialSecurity = weeklyPay * sst;
federalIncome = weeklyPay * fet;
netPay = weeklyPay - socialSecurity - federalIncome;
netpayD = weeklyPay - socialSecurity - federalIncome - dep3;
cout << "Your net weekly pay is " << weeklyPay << endl;
cout << "with $" << socialSecurity << " paid for Social Security,\n";
cout << "$" << federalIncome << " paid for Federal Income Tax,\n"
cout << "$" << union << " paid for union dues,\n";
if (numberDependents >= 3) {
cout << "$" << dep3 << " paid for health insurance for your dependents,\n";
cout << "Leaving you with a net total of $" << netpayD << " for the week.\n";
}
else
cout << "Leaving you with a net total of $" << netPay << " for the week.\n";
return(0);
}
and the compiler errors are:
bookProg6.cpp: In function ‘int main()’:
bookProg6.cpp:8: error: expected primary-expression before ‘int’
bookProg6.cpp:8: error: expected `;' before ‘int’
bookProg6.cpp:31: error: expected primary-expression before ‘union’
bookProg6.cpp:31: error: expected `;' before ‘union’
I don't understand what it's asking me for. I googled those compiler errors, and none of the results really have any relevance to my problem. Any help guys?
|
#
¿
Mar 12, 2008 19:51
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 7, 2024 07:48
|
|
- lmao zebong
- Nov 25, 2006
-
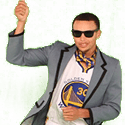
NBA All-Injury First Team
|
FigBug posted:
union is a keyword, you can't use it for a variable name.
you are missing a semicolon on line 28
oh poo poo, thanks a lot! that fixed everything.
I originally had a semicolon there, but I guess I was tweaking the code trying to get union to work and I guess I accidentally deleted it.
|
#
¿
Mar 12, 2008 20:12
|
|
- lmao zebong
- Nov 25, 2006
-
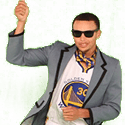
NBA All-Injury First Team
|
I'm still pretty new to C++ (first-year student), and I have some quick questions for a personal program of mine.
I'm writing a program that will randomly generate a number between 1 and 20 for a specified number of players, and then team up the players based on what number they got. The pairings are based on highest number is teamed up with lowest number, and the two middle numbers are paired together. How would I go about doing this? I can code most of the program just fine, but don't really have the programming knowledge to do the 'teaming up' aspect of the program.
Also, after generating these random numbers, I need to set the order of who goes first, based on how biggest number to smallest. How could I do that?
But, just to complicate things up a bit, the teams must alternate on turns. For example, if the two players on team one gets a 20 and a 19, and team two gets a 1 and a 2, then the turns must go team one, then team two, then team one again, even though team one got both the highest numbers. I'm still fairly new when it comes to programming, so help is very much appreciated.
|
#
¿
Mar 31, 2008 08:37
|
|
- lmao zebong
- Nov 25, 2006
-
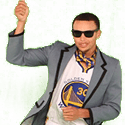
NBA All-Injury First Team
|
ShoulderDaemon posted:

Thanks a lot for the help. I thought that I had this program under control, but now looking at your post I see that there is a lot more going on that I previously expected. Let me try to write some 'pseudocode' for you to explain how I'm thinking it should work.
code:Program asks how many people are playing.
If there are 4 players, ask if if it is a team game or no teams.
Each player rolls a dice to see what number they get.
If a player rolls the same number as another player, they both re-roll.
Teams are assigned based on (lowest, highest) and (medium, medium).
Order of turns is assigned based on highest roll to lowest roll.
I hope that is enough information about what I'm trying to do. I don't want the program to go any further, this is just so me and my friends can easily assign teams for a game we play without going through the motions of rolling actual dice.
The output that I'm looking for (if it was a 4 player game with teams) would simply be:
code:Enter the number of players: 4
Is this a team game or free-for-all?
Enter T for team game, or enter F for free-for-all: T
Player 1 rolled a 2!
Player 2 rolled a 14!
Player 3 rolled a 20!
Player 4 rolled a 7!
Team 1: Player 3 and Player 1
Team 2: Player 2 and Player 4
Order of turns is: Player 3, Player 2, Player 4, Player 1
I understand now what you mean about not looking at the 'bigger picture' of the program. When I was trying to think out this program before I got to a computer, I was just focusing on the smaller parts of the program, like rolling the dice, and when I finally got to a computer realized I was stuck because I don't know how to do the other aspects of the program. I've never used the rand( ) function before, so I don't really know how to access the number it will spit out and use that to set up teams, and order of turns.
|
#
¿
Mar 31, 2008 18:59
|
|
- lmao zebong
- Nov 25, 2006
-
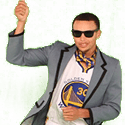
NBA All-Injury First Team
|
Thanks for those links. I was a bit confused as to how the rand function worked, but these references really helped. I'll be sure to bookmark that website if I have any other questions about other aspects of C++.
ShoulderDaemon posted:
code:#include <assert.h>
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main ( int argc, char *argv[] ) {
int ret;
int players;
int teams;
// Prepare the random number generator by seeding with the time.
srandom( time( NULL ) );
// Ask how many players are involved.
fprintf( stdout, "How many players total? " );
ret = fscanf( stdin, "%i\n", &players );
assert( ret == 1 );
assert( players <= 20 ); // If there are more than 20 players, then
// we will never not have duplicate rolls.
// Ask if this is a team game.
// Teams only make sense with an even number of players greater than 2.
if ( players > 2 && players % 2 == 0 ) {
char yesno;
do {
fprintf( stdout, "Is this a team game? (y/n) " );
ret = fscanf( stdin, "%c\n", &yesno );
} while ( yesno != 'y' && yesno != 'Y' && yesno != 'n' && yesno != 'N' );
if ( yesno == 'y' || yesno == 'Y' ) {
teams = 1;
} else {
teams = 0;
};
} else {
teams = 0;
};
int die[players];
// This die-rolling logic is a little bit complicated...
// I'm trying to preserve exactly the "if two people have the same roll,
// then only they reroll" behaviour.
// First, I set each die as "not-yet-rolled", for which I use the value -1.
for ( int i = 0; i < players; ++i )
die[i] = -1;
// Now, for each player, I roll a die...
for ( int i = 0; i < players; ++i ) {
if ( die[i] == -1 ) {
// This player needs to roll.
die[i] = random( ) % 20;
fprintf( stdout, "Player %i rolled %i.\n", i + 1, die[i] + 1 );
};
// Check to see if this roll is the same as any other roll before it.
for ( int j = 0; j < i; ++j ) {
if ( die[i] == die[j] ) {
// These players rolled the same, so they both need to reroll.
fprintf( stdout, "Player %i has the same roll as player %i, so they both reroll.\n", i + 1, j + 1 );
// This is probably the subtlest part of the code I've written.
die[i] = -1;
die[j] = -1;
i = j - 1;
break; // Goes back to the outer loop.
};
};
};
// Print out the order of the players.
// This would be better done by sorting a list of the players;
// the algorithm I have here is equivalent to a bubble sort, but I'm
// using it because it's simple and speed probably doesn't matter too much.
// This is marginally subtle and kind of fun to prove why it works.
int highest_score = 20; // Die rolls are 0..19, so this is higher than any of them.
// This is a loop over play positions.
for ( int i = 0; i < players; ++i ) {
int next_highest_score = -1;
int next_player;
// And this is a loop over players.
for ( int j = 0; j < players; ++j ) {
// We are searching for the player with the largest die roll that is
// still smaller than highest_score.
if ( die[j] < highest_score && die[j] > next_highest_score ) {
next_highest_score = die[j];
next_player = j;
};
};
fprintf( stdout, "Position %i: Player %i\n", i + 1, next_player + 1 );
highest_score = next_highest_score;
};
// Print out the teams, if we're in team play mode.
if ( teams ) {
// The algorithm here mangles the die array,
// which is why I'm doing it after printing the turn order.
// For each team, I'm going to select the players with the highest and lowest
// rolls that haven't yet been chosen. This loop is over teams.
for ( int i = 0; i < players / 2; ++i ) {
int lowest_player, lowest_roll;
int highest_player, highest_roll;
// Out of bounds values.
lowest_roll = 20;
highest_roll = -1;
// And this loop is over players.
for ( int j = 0; j < players; ++j ) {
// If this player hasn't yet been chosen...
if ( die[j] != -1 ) {
// Check if they are the highest roll we've seen so far.
if ( die[j] > highest_roll ) {
highest_roll = die[j];
highest_player = j;
};
// Check if they are the lowest roll we've seen so far.
if ( die[j] < lowest_roll ) {
lowest_roll = die[j];
lowest_player = j;
};
};
};
// Mark the two players as chosen.
die[highest_player] = -1;
die[lowest_player] = -1;
fprintf( stdout, "Team %i: Player %i and Player %i\n", i + 1, highest_player + 1, lowest_player + 1 );
};
};
return 0;
}
Holy poo poo, after looking over this code I understand (vaguely) what is happening and what is going on in each section of the code, but I definitely do not have the programming skill to actually write a program like this. Thanks a lot for the help and discussion guys, but it looks like I'm going to have to scrap this project until I know more. Once I think I have the skills to actually write this program, then I'll for sure speak up again if I need some help. This thread is a great resource, and I enjoy keeping up with this thread (and this subforum in general).
|
#
¿
Mar 31, 2008 22:57
|
|
- lmao zebong
- Nov 25, 2006
-
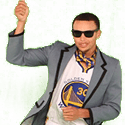
NBA All-Injury First Team
|
I just have a quick question about this programming assignment I've been working on for the last couple days. I'm still entry level, so bare with me if my error is something glaringly obvious.
I'll quote the assignment from my professor's website:
quote:
The birthday paradox is that there is a surprisingly high probability that two people in the same room happen to share the same birthday. By birthday, we mean the same day of the year (ignoring leap years), but not the exact birthday including the birth year or time of day. Write a program that approximates the probability that two people in the same room have the same birthday, for 2 to 50 people in the room.
The program should use simulation to approximate the answer. Over many trials (say, 10,000), randomly assign birthdays to everyone in the room. Count up the number of times at least two people have the same birthday on a trial, and then divide by the number of trials to get an estimated probability that two people share the same birthday for a given room size.
Hint: Try writing a function that can search through an array for a target value, but only search up to a specified number of people.
And here is my code: http://pastebin.com/m40ca99c4
I'm almost positive that my main function is right, and something is going wrong in SameBirthday.
Here is an example of the output I'm getting:
code:For 2 people, the probability of two birthdays is about 0.0052
For 3 people, the probability of two birthdays is about 0.0168
For 4 people, the probability of two birthdays is about 0.0496
For 5 people, the probability of two birthdays is about 0.0445
For 6 people, the probability of two birthdays is about 0.0756
For 7 people, the probability of two birthdays is about 0.1148
For 8 people, the probability of two birthdays is about 0.1688
For 9 people, the probability of two birthdays is about 0.1944
For 10 people, the probability of two birthdays is about 0.239
For 11 people, the probability of two birthdays is about 0.2717
For 12 people, the probability of two birthdays is about 0.3336
For 13 people, the probability of two birthdays is about 0.4498
For 14 people, the probability of two birthdays is about 0.4858
For 15 people, the probability of two birthdays is about 0.5385
For 16 people, the probability of two birthdays is about 0.6576
For 17 people, the probability of two birthdays is about 0.8024
For 18 people, the probability of two birthdays is about 0.8226
For 19 people, the probability of two birthdays is about 0.912
For 20 people, the probability of two birthdays is about 0.962
For 21 people, the probability of two birthdays is about 1.1088
For 22 people, the probability of two birthdays is about 1.2232
For 23 people, the probability of two birthdays is about 1.4007
For 24 people, the probability of two birthdays is about 1.4928
For 25 people, the probability of two birthdays is about 1.5575
For 26 people, the probability of two birthdays is about 1.8096
For 27 people, the probability of two birthdays is about 1.8414
For 28 people, the probability of two birthdays is about 2.044
For 29 people, the probability of two birthdays is about 2.0619
For 30 people, the probability of two birthdays is about 2.322
For 31 people, the probability of two birthdays is about 2.4707
For 32 people, the probability of two birthdays is about 2.5824
For 33 people, the probability of two birthdays is about 2.805
For 34 people, the probability of two birthdays is about 2.9954
For 35 people, the probability of two birthdays is about 3.248
For 36 people, the probability of two birthdays is about 3.1032
For 37 people, the probability of two birthdays is about 3.2782
For 38 people, the probability of two birthdays is about 3.6556
For 39 people, the probability of two birthdays is about 3.9741
For 40 people, the probability of two birthdays is about 3.948
For 41 people, the probability of two birthdays is about 4.2148
For 42 people, the probability of two birthdays is about 4.4772
For 43 people, the probability of two birthdays is about 4.6354
For 44 people, the probability of two birthdays is about 5.1172
For 45 people, the probability of two birthdays is about 5.229
For 46 people, the probability of two birthdays is about 5.566
For 47 people, the probability of two birthdays is about 5.5695
For 48 people, the probability of two birthdays is about 5.6208
For 49 people, the probability of two birthdays is about 5.6742
For 50 people, the probability of two birthdays is about 6.235
Now, the probability shouldn't get above one at all. Why is it getting up to around 6? I'm stumped and have been staring at my code for a while trying to figure out what is going wrong.
Thanks in advance.
|
#
¿
May 12, 2008 02:32
|
|
- lmao zebong
- Nov 25, 2006
-
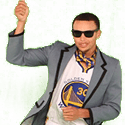
NBA All-Injury First Team
|
Thanks for the help guys. I realized I'm missing a couple braces, which is throwing off the whole thing.
csammis posted:
The problem's in the loops in main(). You're incrementing num_matches multiple times per trial. It only needs to be incremented once per trial, because once two people share the same birthday, the trial is a match and you can stop checking.
I'm not really getting at what you're saying. Am I formatting my loops wrong? I realized I was missing a bracket in the inner loop that calls SameBirthday, and now I have this:
code:// Run trials to see if people have the same birthday
// reset number of matches
for (people=2; people<=50; people++)
{
numMatches = 0;
for(trial = 0; trial <= NUM_TRIALS; trial++)
{
for(i = 0; i < people; i++)
birthdays[i] = (rand( ) % 365) + 1;
for(int y = 0; y < people; y++)
{
if(SameBirthday(birthdays, people))
++numMatches;
}
}
cout << "For " << people << " people, the probability of two " <<
"birthdays is about " <<
static_cast<double>(numMatches) / NUM_TRIALS << endl;
}
}
Shouldn't that only increase numMatches when SameBirthday returns true? Now my output isn't going above one, it's hovering around 0.1.
Sorry for needing all this help guys. I blame me having all this trouble on final stress and overworking myself on classes that have nothing to do with my major. Hooray General Ed!
|
#
¿
May 12, 2008 08:46
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 7, 2024 07:48
|
|
- lmao zebong
- Nov 25, 2006
-
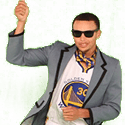
NBA All-Injury First Team
|
Ah I see! That makes sense, and now it seems that my program is working correctly. Thanks a lot for the help guys.
|
#
¿
May 12, 2008 09:15
|
|