- icantfindaname
- Jul 1, 2008
-
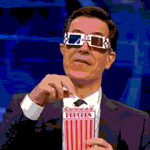
|
Hey, I can't for the life of me figure out what's wrong with this program. It's supposed to take a two dimensional array and find the elements that are larger than their neighbors
code:#include <stdio.h>
#define size 3
int IsLarger (int array[size + 2][size + 2], int i, int j);
main ()
{
srand( (unsigned)time( NULL ) );
int array[size+2][size+2], i, j;
for (i = 0; i < size + 2; i++) {
for (j = 0; j < size + 2; j++) {
array[i][j] = -32766;
}
}
for (i = 1; i <= size; i++) {
for (j = 1; j <= size; j++) {
printf("\nPlease enter an integer: ");
scanf("%d", &array[i][j]);
}
}
for (i = 1; i <= size; i++) {
for(j = 1; j <= size; j++) {
if (IsLarger(array, i, j) == 1) printf ("%d in location (%d, %d)\n", array[i][j], i, j);
}
}
for (i = 1; i <= size; i++) {
for(j = 1; j <= size; j++) {
printf ("[%d]", array[i][j]);
}
printf("\n");
}
}
int IsLarger (int array[size + 2][size + 2], int i, int j)
{
int k, l;
i--;
j--;
for (k = 2; k >= 0; k--) {
for (l = 2; l >= 0; l--) {
if (k && l) break;
if (array[i + k][j + l] >= array[i][j]) return 0;
}
}
return 1;
}
|
#
¿
Dec 21, 2010 23:09
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 17, 2024 13:47
|
|
- icantfindaname
- Jul 1, 2008
-
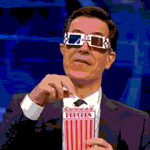
|
Whoops, actually it compiles but doesn't display the
code:printf ("%d in location (%d, %d)\n", array[i][j], i, j);
part, as if IsLarger never returns true
Any more insight?
Here's the program as it is now
code:#include <stdio.h>
#include <stdlib.h>
#define SIZE 3
int IsLarger (int array[SIZE + 2][SIZE + 2], int i, int j);
int main ()
{
int array[SIZE+2][SIZE+2], i, j;
for (i = 0; i < SIZE + 2; i++) {
for (j = 0; j < SIZE + 2; j++) {
array[i][j] = -32766;
}
}
for (i = 1; i <= SIZE; i++) {
for (j = 1; j <= SIZE; j++) {
printf("\nPlease enter an integer: ");
scanf("%d", &array[i][j]);
}
}
for (i = 1; i <= SIZE; i++) {
for(j = 1; j <= SIZE; j++) {
if (IsLarger(array, i, j) == 1) printf ("%d in location (%d, %d)\n", array[i][j], i, j);
}
}
for (i = 1; i <= SIZE; i++) {
for(j = 1; j <= SIZE; j++) {
printf ("[%d]", array[i][j]);
}
printf("\n");
}
system("pause");
}
int IsLarger (int array[SIZE + 2][SIZE + 2], int i, int j)
{
int k, l;
i--;
j--;
for (k = 2; k >= 0; k--)
{
for (l = 2; l >= 0; l--)
{
if ((k != 1) && (l != 1)) {
if (array[i + k][j + l] >= array[i][j]) return 0;
}
}
}
return 1;
}
|
#
¿
Dec 22, 2010 03:32
|
|
- icantfindaname
- Jul 1, 2008
-
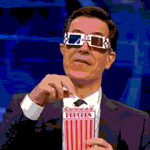
|
MutantBlue posted:
Those line numbers (9,10,11,...) aren't actually in your source file are they? I get similar errors if I don't edit out the line numbers.
I added them to show the lines in the error messages. On second thought, here's the whole program
code:// quicksort.cpp : main project file.
#include "stdafx.h"
#include <stdio.h>
#include "simpio.h"
#define length 16
void sort (int *array1, int length)
{
quick (array1, array1 + length - 1);
}
void quick (int *left, int *right)
{
if (right > left) {
int pivot = left [(right - left) / 2];
int *r = right;
int *l = left;
while (1) {
while (*l < pivot) l++;
while (*r > pivot) r--;
if (l <= r) {
int t = *l;
*l++ = *r;
*r-- = t;
}
if (l > r) break;
}
quick (left, r);
quick (l, right);
}
}
int main (void)
{
int i;
int array1 [length];
printf ("Enter %d elements seperately: ", length);
for (i = 0; i < length; i++) {
array1 [i] = GetInteger();
}
for (i = 0; i < length; i++) printf ("[%d]", array1[i]);
printf ("\n");
sort (array1, length);
for (i = 0; i < length; i++) printf ("[%d]", array1[i]);
printf ("\n");
getchar ();
}
Sorry about that
|
#
¿
Mar 22, 2011 03:30
|
|
- icantfindaname
- Jul 1, 2008
-
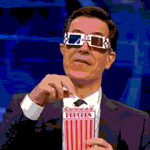
|
So I have this basic file i/o program, but when it runs I get a segmentation fault (and also a compiler warning about exit()). I'm running x64 Ubuntu. What exactly is the problem? Both in.list and out.list exist and are in the same directory as the binary.
code:#include <stdio.h>
int main(int argc, char **argv)
{
FILE *ifp, *ofp;
char outputFilename[] = "out.list";
char username[9];
int score;
ifp = fopen("in.list", "r");
if (ifp == NULL)
{
printf("Can't open input file in.list!\n");
exit(1);
}
ofp = fopen("out.list", "w");
if (ofp = NULL)
{
fprintf(stderr, "Can't open output file %s!\n", outputFilename);
printf("DONGS");
exit(1);
}
while (fscanf(ifp, "%s %d", username, &score) != EOF)
{
fprintf(ofp, "%s %d\n", username, score+10);
}
fclose(ofp);
fclose(ifp);
return 0;
}
edit: Including stdlib fixes the error, but the segmentation fault is still there.
icantfindaname fucked around with this message at 22:23 on Dec 22, 2012
|
#
¿
Dec 22, 2012 22:13
|
|
- icantfindaname
- Jul 1, 2008
-
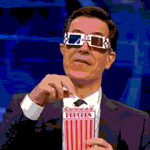
|
edit: Okay, I fixed the problem. But I'm still interested in what the problem actually was. The string behavior is loving bizarre: When I try to append one string to another it's just replacing the first X characters of the first string with the second. Removing the final character of the first string fixes it but what is that character doing even?
code:#include <iostream>
#include <string>
#include <fstream>
#include <sstream>
#include "BinaryStringTree.h"
int main ()
{
BinaryStringTree *tree = new BinaryStringTree();
std::ifstream input;
std::string line, first, last;
input.open ("hash_short");
if (input.is_open ()) {
while (std::getline (input, line)) {
// std::cout << line << std::endl;
int i = 0;
while (i < line.size()) {
if (line[i] == '#') break;
i++;
}
last = line.substr (i + 1, std::string::npos);
first = line.substr (0, i + 1);
std::cout << first << std::endl << last << std::endl;
last.append (first);
std::cout << last << std::endl;
tree->insertNode (last);
}
input.close ();
}
// std::cout << "\nIn-order traversal of this tree: \n";
// std::cout << std::endl;
// tree->getInOrderTraversal (tree);
// std::cout << std::endl;
return 0;
}
Output:
code:1, #
yapbozmeb
1, #ozmeb
2, #
gameinsight
2, #insight
3, #
android
3, #oid
4, #
rt
4, #
5, #
premiosgoya
5, #iosgoya
6, #
androidgames
6, #oidgames
7, #
goya2014
7, #2014
8, #
ipad
8, #
9, #
ipadgames
9, #games
10, #
birnesilgeliyor
10, #silgeliyor
icantfindaname fucked around with this message at 08:09 on Nov 26, 2015
|
#
¿
Nov 26, 2015 00:41
|
|
- icantfindaname
- Jul 1, 2008
-
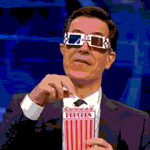
|
I'm trying to write a program in C that parses a string containing 5 currencies and converts them to dollars, character by character with getchar(), so for example the input "$500£500¥500€500₹500"
What I have works correctly except for a bizarre bug where the value after the amount in pounds always shows up zero, so the above input gives this output
code:$500
$823 in sterling
$0 in yen
$683 in euros
$8 in rupees
The input $500£500$500 gives
code:$500
$823 in sterling
$0
My code is this. I use a C string to hold the characters grabbed from stdin before converting them to an integer, and if you print the raw string containing the characters supposed to be $500 in the above it's super hosed up, the characters themselves seem to be random numbers. What the heck is the problem?
code:#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char * append(char *str, char c)
{
size_t len = strlen(str);
char *str2 = malloc(len + 1 + 1);
strcpy(str2, str);
str2[len] = c;
str2[len+1] = '\0';
free(str);
return str2;
}
int main ()
{
int n, total = 0;
char *str = malloc(sizeof(char));
printf("Please enter a sum of money in sterling, yen, rupees or euros: ");
for (int c = getchar(); c != '\n' && c != EOF; c = getchar()) {
if (c == 0x24) {
while ((c = getchar()) != '\n' && c != EOF) {
if (c == 0xC2 || c == 0xE2) { ungetc(c, stdin); break; }
str = append(str, c);
}
n = atoi(str);
printf("%d %d %d\n", str[0], str[1], str[2]);
printf("$%d\n", n);
total += n;
free(str);
str = malloc(sizeof(char));
continue;
}
if (c == 0xC2) {
c = getchar();
if (c == 0xA3) {
while ((c = getchar()) != '\n' && c != EOF) {
if (c == 0x24 || c == 0xE2 || c == 0xC2) { ungetc(c, stdin); break; }
str = append(str, c);
}
n = atoi(str);
n = n * 1000 / 607;
printf("$%d in sterling\n", n);
total += n;
free(str);
str = malloc(sizeof(char));
continue;
}
if (c == 0xA5) {
while ((c = getchar()) != '\n' && c != EOF) {
if (c == 0x24 || c == 0xE2 || c == 0xC2) { ungetc(c, stdin); break; }
str = append(str, c);
}
n = atoi(str);
n = n * 100 / 10404;
printf("$%d in yen\n", n);
total += n;
str = malloc(sizeof(char));
continue;
}
}
if (c == 0xE2) {
c = getchar();
c = getchar();
if (c == 0xAC) {
while ((c = getchar()) != '\n' && c != EOF) {
if (c == 0x24 || c == 0xC2 || c == 0xE2) { ungetc(c, stdin); break; }
str = append(str, c);
}
n = atoi(str);
n = n * 1000 / 732;
printf("$%d in euros\n", n);
total += n;
str = malloc(sizeof(char));
continue;
}
if (c == 0xB9) {
while ((c = getchar()) != '\n' && c != EOF) {
if (c == 0x24 || c == 0xC2 || c == 0xE2) { ungetc(c, stdin); break; }
str = append(str, c);
}
n = atoi(str);
n = n * 1000 / 61903;
printf("$%d in rupees\n", n);
total += n;
str = malloc(sizeof(char));
continue;
}
}
}
free(str);
return 0;
}
|
#
¿
Dec 29, 2016 02:58
|
|
- icantfindaname
- Jul 1, 2008
-
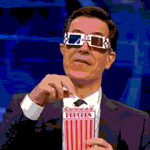
|
I’m trying to do these SDL tutorials in Ubuntu and the programs won’t compile, when I try to compile this file it gives this error
https://lazyfoo.net/tutorials/SDL/15_rotation_and_flipping/index.php
https://pastebin.com/HsGLyTEh
code:VirtualBox:~/sdl/tutorial6/15_rotation_and_flipping$ gcc 15_ro tation_and_flipping.cpp -w -lSDL2 -lSDL2_image -I/usr/local/include/SDL2 -D_REEN TRANT
/usr/bin/ld: /tmp/ccldwsPN.o: in function `LTexture::loadFromFile(std::__cxx11:: basic_string<char, std::char_traits<char>, std::allocator<char> >)':
15_rotation_and_flipping.cpp:(.text+0x81): undefined reference to `std::__cxx11: :basic_string<char, std::char_traits<char>, std::allocator<char> >::c_str() cons t'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0xa8): undefined reference to ` std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> >: :c_str() const'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x12b): undefined reference to `std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> > ::c_str() const'
/usr/bin/ld: /tmp/ccldwsPN.o: in function `loadMedia()':
15_rotation_and_flipping.cpp:(.text+0x4f8): undefined reference to `std::allocat or<char>::allocator()'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x50f): undefined reference to `std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> > ::basic_string(char const*, std::allocator<char> const&)'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x533): undefined reference to `std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> > ::~basic_string()'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x53f): undefined reference to `std::allocator<char>::~allocator()'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x57b): undefined reference to `std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> > ::~basic_string()'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x590): undefined reference to `std::allocator<char>::~allocator()'
/usr/bin/ld: /tmp/ccldwsPN.o:(.data.rel.local.DW.ref.__gxx_personality_v0[DW.ref .__gxx_personality_v0]+0x0): undefined reference to `__gxx_personality_v0'
collect2: error: ld returned 1 exit status
Some research says this might be caused by the SDL libraries being compiled with a different version of GCC, but I reinstalled SDL from source and it still gives the same exact error
Specifying std=c++11 does not help either
icantfindaname fucked around with this message at 02:39 on Feb 10, 2021
|
#
¿
Feb 10, 2021 02:36
|
|
- icantfindaname
- Jul 1, 2008
-
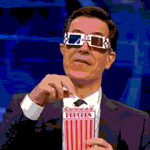
|
Now I’m getting this error. I have the packages for libsdl2-image and libsdl2-image-dev both installed, but it doesn’t seem to recognize them. There is indeed a prototype for IMG_Load() in /usr/include/SDL2/SDL_image.h
https://pastebin.com/eiEkCJ8E
code:VirtualBox:~/SDL/tutorial15/15_rotation_and_flipping$ g++ -lSDL2 -lSDL2_image -I/usr/include/SDL2 -D_REENTRANT 15_rotation_and_flipping.cpp
/usr/bin/ld: /tmp/ccxrAWJZ.o: in function `LTexture::loadFromFile(std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> >)':
15_rotation_and_flipping.cpp:(.text+0x89): undefined reference to `IMG_Load'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x99): undefined reference to `SDL_GetError'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0xe3): undefined reference to `SDL_MapRGB'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0xf6): undefined reference to `SDL_SetColorKey'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x10c): undefined reference to `SDL_CreateTextureFromSurface'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x11c): undefined reference to `SDL_GetError'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x16c): undefined reference to `SDL_FreeSurface'
/usr/bin/ld: /tmp/ccxrAWJZ.o: in function `LTexture::free()':
15_rotation_and_flipping.cpp:(.text+0x1b7): undefined reference to `SDL_DestroyTexture'
/usr/bin/ld: /tmp/ccxrAWJZ.o: in function `LTexture::setColor(unsigned char, unsigned char, unsigned char)':
15_rotation_and_flipping.cpp:(.text+0x214): undefined reference to `SDL_SetTextureColorMod'
/usr/bin/ld: /tmp/ccxrAWJZ.o: in function `LTexture::setBlendMode(SDL_BlendMode)':
15_rotation_and_flipping.cpp:(.text+0x23f): undefined reference to `SDL_SetTextureBlendMode'
/usr/bin/ld: /tmp/ccxrAWJZ.o: in function `LTexture::setAlpha(unsigned char)':
15_rotation_and_flipping.cpp:(.text+0x26c): undefined reference to `SDL_SetTextureAlphaMod'
/usr/bin/ld: /tmp/ccxrAWJZ.o: in function `LTexture::render(int, int, SDL_Rect*, double, SDL_Point*, SDL_RendererFlip)':
15_rotation_and_flipping.cpp:(.text+0x310): undefined reference to `SDL_RenderCopyEx'
/usr/bin/ld: /tmp/ccxrAWJZ.o: in function `init()':
15_rotation_and_flipping.cpp:(.text+0x36d): undefined reference to `SDL_Init'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x379): undefined reference to `SDL_GetError'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x3a9): undefined reference to `SDL_SetHint'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x3ea): undefined reference to `SDL_CreateWindow'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x402): undefined reference to `SDL_GetError'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x438): undefined reference to `SDL_CreateRenderer'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x450): undefined reference to `SDL_GetError'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x48e): undefined reference to `SDL_SetRenderDrawColor'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x49f): undefined reference to `IMG_Init'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x4b0): undefined reference to `SDL_GetError'
/usr/bin/ld: /tmp/ccxrAWJZ.o: in function `close()':
15_rotation_and_flipping.cpp:(.text+0x5cc): undefined reference to `SDL_DestroyRenderer'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x5db): undefined reference to `SDL_DestroyWindow'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x5f6): undefined reference to `IMG_Quit'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x5fb): undefined reference to `SDL_Quit'
/usr/bin/ld: /tmp/ccxrAWJZ.o: in function `main':
15_rotation_and_flipping.cpp:(.text+0x685): undefined reference to `SDL_PollEvent'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x74d): undefined reference to `SDL_SetRenderDrawColor'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x75c): undefined reference to `SDL_RenderClear'
/usr/bin/ld: 15_rotation_and_flipping.cpp:(.text+0x7d3): undefined reference to `SDL_RenderPresent'
collect2: error: ld returned 1 exit status
|
#
¿
Feb 10, 2021 04:44
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 17, 2024 13:47
|
|