- FastEddie
- Oct 4, 2003
-
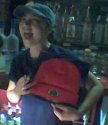
|
Remulak posted:
You're right. They're what's called a logical shift. If it carried the bit around it would be a rotate.
No, they're bitwise shifts, because they operate on bits, rather than the boolean interpretation of the collections of bits. I have no idea what a logical shift would be.
|
#
¿
Feb 17, 2008 05:56
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 6, 2024 08:53
|
|
- FastEddie
- Oct 4, 2003
-
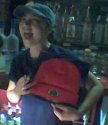
|
code:void reverse_string(char *sz) {
char *e;
if (!sz[0]) return;
for (e = sz; e[1]; ++e);
for (; sz < e; ++sz, --e) {
char t = *e;
*e = *sz;
*sz = t;
}
}
And here's a test:code:#include <stdio.h>
#include <string.h>
int errors = 0;
void test(const char *in, const char *expected) {
char *in_copy = strdup(in);
reverse_string(in_copy);
if (strcmp(expected, in_copy)) {
printf("FAIL - expected %s, got %s\n", expected, in_copy);
++errors;
}
free(in_copy);
}
int main() {
test("", "");
test("a", "a");
test("abc", "cba");
test("Hello, World !", "! dlroW ,olleH");
if (!errors) puts("PASS");
return errors;
}
|
#
¿
Dec 8, 2009 08:56
|
|
- FastEddie
- Oct 4, 2003
-
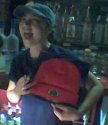
|
Avenging Dentist posted:
I hereby present FastEddie the Cavern of COBOL FizzBuzz Award for correctly solving the wrong problem!!!
Not if you put "olleh dneirf" into it. He wants to reverse the words in a sentence, no? And he's already reversed each word.
|
#
¿
Dec 9, 2009 00:37
|
|
- FastEddie
- Oct 4, 2003
-
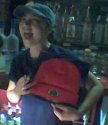
|
Avenging Dentist posted:
Yes, that is clearly what he meant and you're clearly not just backpedaling in an attempt to save face.
Why would he want to reverse the characters in each word? That's the stupidest interview question I've ever heard of!
|
#
¿
Dec 9, 2009 00:45
|
|
- FastEddie
- Oct 4, 2003
-
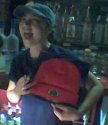
|
irishcoffee posted:
I have a function definition as follows:
void foo(void *arr, size_t num_elem, size_t elem_size)
Arr can point to any array of strings, ints, longs, floats, whatever.
How would I go about memcpying this array regardless of type
code:void *destination = ...
memcpy(destination, arr, num_elem * elem_size);
quote:and referencing its elements, regardless of type.
code:void *elem = (char*)arr + (index * elem_size);
You'll need another cast if you're using C++.
FastEddie fucked around with this message at 21:01 on Dec 9, 2009
|
#
¿
Dec 9, 2009 20:58
|
|
- FastEddie
- Oct 4, 2003
-
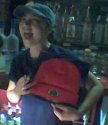
|
Awesome Andrew posted:
Is it possible to pass a single (and not predefined) element of a structure to a parameter?
For instance:
code:struct Stats {
int health, mana;
int strength, vitality, agility;
int intelligence, willpower;
};
code:class Character : GameObject {
...
Stats base_stats;
public:
...
void change_stat(std::string stat_name, int magnitude) {
base_stats.stat_name =+ magnitude;
}
...
};
Or do I just need to create a separate function for each element?
You could use an array of integers in the struct, and then have an enum for indexing into the array.code:enum StatType {
HEALTH, MANA, VITALITY, ETC,
NUM_STATS;
};
struct Stats {
int value[NUM_STATS];
};
Then instead of passing a reference or pointer, you'd pass the enum for the stat you wanted to update.code:void change_stat(StatType stat, int magnitude) {
base_stats.value[stat] += magnitude;
}
And then you'd have something like this:code:obj.change_stat(HEALTH, 10);
|
#
¿
Apr 11, 2011 01:27
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 6, 2024 08:53
|
|