- Whilst farting I
- Apr 25, 2006
-
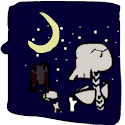
|
I'm writing an applet which plays a card game, but I wrote it misunderstanding how the game works. How it's supposed to work: three cards are drawn, two are put face up, user enters how much money he/she would like to bet that the integer value of the third card is between the two being shown, third card is shown and winnings are added/subtracted accordingly. How I wrote it: user enters how much money they'd like to bet, all 3 cards are drawn at once and uses whatever bet was given before beginning the round.
beginRound is the function that compares the cards, determines if the card is in between the first and second card drawn, and accordingly adds/subtracts from the user's total winnings.
The user enters into a textfield his/her bet and any button clicked will automatically get the bet for the user, but how do I make it so for begin round, two cards are drawn, and then have the program wait for the user to enter a new bet and click the corresponding button associated with bet before continuing with the rest of the function? I feel like right after the line with curCard3 is where it should wait for the user's bet before continuing, but I can't find any information on how to make the program wait on a non-input dialog basis. The rest of the program works perfectly. Thank you!
code:public void actionPerformed(ActionEvent e)
{
// Gets the user's bet no matter what action is called
int currentBet = getBet();
// Starts the round, calls function to get the winnings
// and appends them
if (e.getActionCommand().equals("startround"))
{
// Deals three cards, first two displayed to user, all removed from deck
curCard1 = deck.dealCard();
curCard2 = deck.dealCard();
curCard3 = deck.dealCard();
newWinnings = beginRound(currentBet, currentWinnings);
currentWinnings = newWinnings;
displayWinnings.setText("" + currentWinnings + "");
}
// Gets the user's bet
if (e.getActionCommand().equals("bet"))
{
currentBet = getBet();
}
// If user wants a new game, erases winnings, clears
// text areas, and creates a new deck
if (e.getActionCommand().equals("newgame"))
{
currentWinnings = 0;
displayWinnings.setText(null);
deck.shuffle();
}
} // end actionPerformed
|
#
¿
Apr 9, 2008 11:04
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 6, 2024 22:46
|
|
- Whilst farting I
- Apr 25, 2006
-
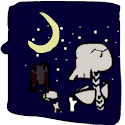
|
triplekungfu posted:
I may be missing something, but shouldn't the flow go something like this:
[list=1]
[*]Display 2 cards
[*]Accept user's bet
[*]Final card and winnings calculation
[/list]
Now, #1 is triggered by "startround", #2 by "bet", and #3 by #2?
You're right, I knew something didn't seem right. I solved the problem by dealing the cards in the start round function and moving the actual comparison and calculation functions and display into the bet.
The GUI includes 3 buttons: Start a New Game (start from scratch), Start A New Round (deals 3 new cards and displays 2), and Bet (gets the bet from the user, displays the last card, and gives output). So the flow is now identical to what you just posted. This is really rough (error-checking statements to be added, variable names are hosed up, graphic display of cards to add), but it works. Thank you!
code:public void actionPerformed(ActionEvent e)
{
int currentBet;
// Deals 3 cards and displays first 2 so user can bet
if (e.getActionCommand().equals("startround"))
{
curCard1 = deck.dealCard();
curCard2 = deck.dealCard();
curCard3 = deck.dealCard();
displayBet.append("Card 1: " + curCard1 + "\nCard 2: " + curCard2 + "\nCard 3: ");
}
// Gets the user's bet after displaying the first two cards
if (e.getActionCommand().equals("bet"))
{
currentBet = getBet();
// Calculates the amount of cards left in the deck
noCardsLeft = deck.cardsLeft();
// Calculates if third card is between first two, appends final card with results
newWinnings = beginRound(currentBet, currentWinnings);
displayBet.append("" + curCard3 + "\n\n" + noCardsLeft + "\n\n");
currentWinnings = newWinnings;
displayWinnings.setText("" + currentWinnings + "");
}
// If user wants a new game, erases winnings, clears
// text areas, and creates a new deck
if (e.getActionCommand().equals("newgame"))
{
currentWinnings = 0;
displayWinnings.setText(null);
deck.shuffle();
}
} // end actionPerformed
Out of curiousity for the future, though, does anyone know a better way for making a program wait on a non-input dialog basis besides the sleep method BELL END mentioned?
|
#
¿
Apr 9, 2008 15:03
|
|