- Adbot
-
ADBOT LOVES YOU
|
|
#
?
Apr 27, 2024 16:45
|
|
- Bruegels Fuckbooks
- Sep 14, 2004
-
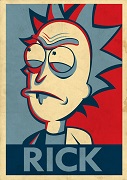
Now, listen - I know the two of you are very different from each other in a lot of ways, but you have to understand that as far as Grandpa's concerned, you're both pieces of shit! Yeah. I can prove it mathematically.
|
This looks like an ideal use case for blockchain. I'll start the wiki.
I think we can do this. Note that the code for peer to peer and proof of work aspects of blockchain is omitted as an exercise for the reader.
code:using System;
using System.Collections.Generic;
using System.Linq;
using System.Security.Cryptography;
using System.Text;
using System.Threading.Tasks;
namespace BlockchainIsEven
{
public class Transaction
{
public string FromAddress { get; set; }
public string ToAddress { get; set; }
public int Amount { get; set; }
public byte[] Signature { get; set; }
public bool Validate()
{
// Perform basic transaction validation
return !string.IsNullOrEmpty(FromAddress) && !string.IsNullOrEmpty(ToAddress) && Amount > 0;
}
}
public class Block
{
public int Index { get; set; }
public DateTime Timestamp { get; set; }
public string PreviousHash { get; set; }
public string Hash { get; set; }
public Transaction[] Transactions { get; set; }
public void Mine(int difficulty)
{
string hashPrefix = new string('0', difficulty);
while (!Hash.StartsWith(hashPrefix))
{
Hash = CalculateHash();
}
}
private string CalculateHash()
{
using (SHA256 sha256 = SHA256.Create())
{
string rawData = $"{Index}{Timestamp}{PreviousHash}{SerializeTransactions()}";
byte[] bytes = Encoding.UTF8.GetBytes(rawData);
byte[] hash = sha256.ComputeHash(bytes);
return BitConverter.ToString(hash).Replace("-", "");
}
}
private string SerializeTransactions()
{
// Serialize transactions to a string representation
return string.Join(",", Transactions);
}
public string CalculateMerkleRoot()
{
var transactionHashes = Transactions.Select(t => CalculateHash(t.ToString())).ToArray();
return BuildMerkleTree(transactionHashes);
}
private string BuildMerkleTree(string[] hashes)
{
if (hashes.Length == 1)
return hashes[0];
var parentHashes = new List<string>();
for (int i = 0; i < hashes.Length; i += 2)
{
if (i + 1 < hashes.Length)
{
var leftHash = hashes[i];
var rightHash = hashes[i + 1];
var combinedHash = CalculateHash($"{leftHash}{rightHash}");
parentHashes.Add(combinedHash);
}
else
{
parentHashes.Add(hashes[i]);
}
}
return BuildMerkleTree(parentHashes.ToArray());
}
private string CalculateHash(string data)
{
using (SHA256 sha256 = SHA256.Create())
{
byte[] bytes = Encoding.UTF8.GetBytes(data);
byte[] hash = sha256.ComputeHash(bytes);
return BitConverter.ToString(hash).Replace("-", "");
}
}
}
public class Blockchain
{
private readonly List<Block> _chain = new List<Block>();
private readonly List<Transaction> _pendingTransactions = new List<Transaction>();
public const int DIFFICULTY = 4;
public const int BLOCK_REWARD = 10;
public const int TRANSACTION_FEE = 1;
public void AddTransaction(Transaction transaction)
{
if (transaction.Validate())
_pendingTransactions.Add(transaction);
}
public async Task MineBlock(string minerAddress)
{
var block = new Block
{
Index = _chain.Count + 1,
Timestamp = DateTime.Now,
PreviousHash = _chain.LastOrDefault()?.Hash ?? string.Empty,
Transactions = _pendingTransactions.ToArray()
};
block.Mine(DIFFICULTY);
_chain.Add(block);
_pendingTransactions.Clear();
var coinbaseTransaction = new Transaction
{
FromAddress = string.Empty,
ToAddress = minerAddress,
Amount = BLOCK_REWARD
};
_pendingTransactions.Add(coinbaseTransaction);
await BroadcastBlock(block);
}
private async Task BroadcastBlock(Block block)
{
var apiClient = new BlockchainAPIClient();
await apiClient.BroadcastBlockAsync(block);
}
public async Task<bool> IsValidChain()
{
for (int i = 1; i < _chain.Count; i++)
{
var currentBlock = _chain[i];
var previousBlock = _chain[i - 1];
if (currentBlock.Hash != currentBlock.CalculateHash())
return false;
if (currentBlock.PreviousHash != previousBlock.Hash)
return false;
var apiClient = new BlockchainAPIClient();
if (!await apiClient.VerifyProofOfWorkAsync(currentBlock, DIFFICULTY))
return false;
}
return true;
}
public async Task ResolveConflicts()
{
var apiClient = new BlockchainAPIClient();
var peers = await apiClient.GetPeersAsync();
var newChain = _chain;
foreach (var peer in peers)
{
var response = await apiClient.GetChainFromPeerAsync(peer);
var chain = response.Chain;
if (chain.Count > newChain.Count && await IsValidChain(chain))
newChain = chain;
}
if (newChain != _chain)
_chain = newChain;
}
}
public interface IIsEvenStrategy
{
Task<bool> IsEvenAsync(int number);
}
public class TraditionalIsEvenStrategy : IIsEvenStrategy
{
public Task<bool> IsEvenAsync(int number)
{
return Task.FromResult(number % 2 == 0);
}
}
public class BlockchainIsEvenStrategy : IIsEvenStrategy
{
private readonly Blockchain _blockchain = new Blockchain();
public async Task<bool> IsEvenAsync(int number)
{
var transaction = CreateTransaction(number);
_blockchain.AddTransaction(transaction);
await _blockchain.MineBlock("miner1");
await _blockchain.ResolveConflicts();
return transaction.Amount % 2 == 0;
}
private Transaction CreateTransaction(int number)
{
var transaction = new Transaction
{
FromAddress = "sender",
ToAddress = "receiver",
Amount = number - Blockchain.TRANSACTION_FEE
};
// Sign the transaction with a dummy private key
using (ECDsa ecdsa = ECDsa.Create())
{
byte[] transactionBytes = Encoding.UTF8.GetBytes(transaction.ToString());
transaction.Signature = ecdsa.SignData(transactionBytes, HashAlgorithmName.SHA256);
}
return transaction;
}
}
public class IsEvenChecker
{
private IIsEvenStrategy _strategy;
public IsEvenChecker(IIsEvenStrategy strategy)
{
_strategy = strategy;
}
public async Task<bool> IsEvenAsync(int number)
{
return await _strategy.IsEvenAsync(number);
}
}
public class Program
{
public static async Task Main(string[] args)
{
int number = 42;
IIsEvenStrategy traditionalStrategy = new TraditionalIsEvenStrategy();
IsEvenChecker traditionalChecker = new IsEvenChecker(traditionalStrategy);
bool isEvenTraditional = await traditionalChecker.IsEvenAsync(number);
Console.WriteLine($"Traditional IsEven: {isEvenTraditional}");
IIsEvenStrategy blockchainStrategy = new BlockchainIsEvenStrategy();
IsEvenChecker blockchainChecker = new IsEvenChecker(blockchainStrategy);
Console.WriteLine("Checking if the number is even using blockchain...");
bool isEvenBlockchain = await blockchainChecker.IsEvenAsync(number);
Console.WriteLine($"Blockchain IsEven: {isEvenBlockchain}");
}
}
}
|
#
?
Mar 13, 2024 01:41
|
|
- Hammerite
- Mar 9, 2007
-
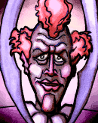
And you don't remember what I said here, either, but it was pompous and stupid.
-
Jade Ear Joe
|
you got chatgpt or something to write all that right? If you sat down and wrote that yourself for the sake of making a joke in the computer programming thread then either you're crazy or i am.
|
#
?
Mar 13, 2024 02:07
|
|
- ShoulderDaemon
- Oct 9, 2003
-
support goon fund
-
Taco Defender
|
I briefly looked into generating a lookup table using templates but that's way too much effort for me.
code:#include <array>
#include <cstdint>
#include <iostream>
#include <utility>
template<size_t i>
struct IsEven {
constexpr static bool isEven() {
return !IsEven<i-1>::isEven();
}
};
template<>
struct IsEven<0> {
constexpr static bool isEven() {
return true;
}
};
static const size_t MAX = 65536;
template<size_t... Is>
constexpr auto precomputeIsEven(std::index_sequence<Is...>) {
return std::array<bool, MAX>{ IsEven<Is>::isEven()... };
}
constexpr auto isEven = precomputeIsEven(std::make_index_sequence<MAX>());
int main(int argc, const char *argv[]) {
for (unsigned int i = 0; i < MAX; ++i)
std::cout << i << " is " << (isEven[i] ? "even" : "odd") << ".\n";
return 0;
}
I have been paid actual money for writing code that does this trick for a "real" problem.
|
#
?
Mar 13, 2024 02:11
|
|
- Bruegels Fuckbooks
- Sep 14, 2004
-
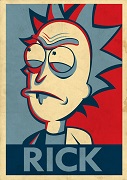
Now, listen - I know the two of you are very different from each other in a lot of ways, but you have to understand that as far as Grandpa's concerned, you're both pieces of shit! Yeah. I can prove it mathematically.
|
you got chatgpt or something to write all that right? If you sat down and wrote that yourself for the sake of making a joke in the computer programming thread then either you're crazy or i am.
I've been testing the new claude model and it's good at programming humor. It doesn't like violence in source code comments so you still need to use chatgpt for any code that involves violence but it's generally more flexible otherwise.
|
#
?
Mar 13, 2024 03:19
|
|
- Volmarias
- Dec 31, 2002
-
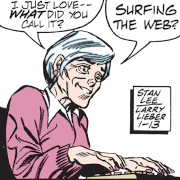
EMAIL... THE INTERNET... SEARCH ENGINES...
|
I think we can do this. Note that the code for peer to peer and proof of work aspects of blockchain is omitted as an exercise for the reader.
code:using System;
using System.Collections.Generic;
using System.Linq;
using System.Security.Cryptography;
using System.Text;
using System.Threading.Tasks;
namespace BlockchainIsEven
{
public class Transaction
{
public string FromAddress { get; set; }
public string ToAddress { get; set; }
public int Amount { get; set; }
public byte[] Signature { get; set; }
public bool Validate()
{
// Perform basic transaction validation
return !string.IsNullOrEmpty(FromAddress) && !string.IsNullOrEmpty(ToAddress) && Amount > 0;
}
}
public class Block
{
public int Index { get; set; }
public DateTime Timestamp { get; set; }
public string PreviousHash { get; set; }
public string Hash { get; set; }
public Transaction[] Transactions { get; set; }
public void Mine(int difficulty)
{
string hashPrefix = new string('0', difficulty);
while (!Hash.StartsWith(hashPrefix))
{
Hash = CalculateHash();
}
}
private string CalculateHash()
{
using (SHA256 sha256 = SHA256.Create())
{
string rawData = $"{Index}{Timestamp}{PreviousHash}{SerializeTransactions()}";
byte[] bytes = Encoding.UTF8.GetBytes(rawData);
byte[] hash = sha256.ComputeHash(bytes);
return BitConverter.ToString(hash).Replace("-", "");
}
}
private string SerializeTransactions()
{
// Serialize transactions to a string representation
return string.Join(",", Transactions);
}
public string CalculateMerkleRoot()
{
var transactionHashes = Transactions.Select(t => CalculateHash(t.ToString())).ToArray();
return BuildMerkleTree(transactionHashes);
}
private string BuildMerkleTree(string[] hashes)
{
if (hashes.Length == 1)
return hashes[0];
var parentHashes = new List<string>();
for (int i = 0; i < hashes.Length; i += 2)
{
if (i + 1 < hashes.Length)
{
var leftHash = hashes[i];
var rightHash = hashes[i + 1];
var combinedHash = CalculateHash($"{leftHash}{rightHash}");
parentHashes.Add(combinedHash);
}
else
{
parentHashes.Add(hashes[i]);
}
}
return BuildMerkleTree(parentHashes.ToArray());
}
private string CalculateHash(string data)
{
using (SHA256 sha256 = SHA256.Create())
{
byte[] bytes = Encoding.UTF8.GetBytes(data);
byte[] hash = sha256.ComputeHash(bytes);
return BitConverter.ToString(hash).Replace("-", "");
}
}
}
public class Blockchain
{
private readonly List<Block> _chain = new List<Block>();
private readonly List<Transaction> _pendingTransactions = new List<Transaction>();
public const int DIFFICULTY = 4;
public const int BLOCK_REWARD = 10;
public const int TRANSACTION_FEE = 1;
public void AddTransaction(Transaction transaction)
{
if (transaction.Validate())
_pendingTransactions.Add(transaction);
}
public async Task MineBlock(string minerAddress)
{
var block = new Block
{
Index = _chain.Count + 1,
Timestamp = DateTime.Now,
PreviousHash = _chain.LastOrDefault()?.Hash ?? string.Empty,
Transactions = _pendingTransactions.ToArray()
};
block.Mine(DIFFICULTY);
_chain.Add(block);
_pendingTransactions.Clear();
var coinbaseTransaction = new Transaction
{
FromAddress = string.Empty,
ToAddress = minerAddress,
Amount = BLOCK_REWARD
};
_pendingTransactions.Add(coinbaseTransaction);
await BroadcastBlock(block);
}
private async Task BroadcastBlock(Block block)
{
var apiClient = new BlockchainAPIClient();
await apiClient.BroadcastBlockAsync(block);
}
public async Task<bool> IsValidChain()
{
for (int i = 1; i < _chain.Count; i++)
{
var currentBlock = _chain[i];
var previousBlock = _chain[i - 1];
if (currentBlock.Hash != currentBlock.CalculateHash())
return false;
if (currentBlock.PreviousHash != previousBlock.Hash)
return false;
var apiClient = new BlockchainAPIClient();
if (!await apiClient.VerifyProofOfWorkAsync(currentBlock, DIFFICULTY))
return false;
}
return true;
}
public async Task ResolveConflicts()
{
var apiClient = new BlockchainAPIClient();
var peers = await apiClient.GetPeersAsync();
var newChain = _chain;
foreach (var peer in peers)
{
var response = await apiClient.GetChainFromPeerAsync(peer);
var chain = response.Chain;
if (chain.Count > newChain.Count && await IsValidChain(chain))
newChain = chain;
}
if (newChain != _chain)
_chain = newChain;
}
}
public interface IIsEvenStrategy
{
Task<bool> IsEvenAsync(int number);
}
public class TraditionalIsEvenStrategy : IIsEvenStrategy
{
public Task<bool> IsEvenAsync(int number)
{
return Task.FromResult(number % 2 == 0);
}
}
public class BlockchainIsEvenStrategy : IIsEvenStrategy
{
private readonly Blockchain _blockchain = new Blockchain();
public async Task<bool> IsEvenAsync(int number)
{
var transaction = CreateTransaction(number);
_blockchain.AddTransaction(transaction);
await _blockchain.MineBlock("miner1");
await _blockchain.ResolveConflicts();
return transaction.Amount % 2 == 0;
}
private Transaction CreateTransaction(int number)
{
var transaction = new Transaction
{
FromAddress = "sender",
ToAddress = "receiver",
Amount = number - Blockchain.TRANSACTION_FEE
};
// Sign the transaction with a dummy private key
using (ECDsa ecdsa = ECDsa.Create())
{
byte[] transactionBytes = Encoding.UTF8.GetBytes(transaction.ToString());
transaction.Signature = ecdsa.SignData(transactionBytes, HashAlgorithmName.SHA256);
}
return transaction;
}
}
public class IsEvenChecker
{
private IIsEvenStrategy _strategy;
public IsEvenChecker(IIsEvenStrategy strategy)
{
_strategy = strategy;
}
public async Task<bool> IsEvenAsync(int number)
{
return await _strategy.IsEvenAsync(number);
}
}
public class Program
{
public static async Task Main(string[] args)
{
int number = 42;
IIsEvenStrategy traditionalStrategy = new TraditionalIsEvenStrategy();
IsEvenChecker traditionalChecker = new IsEvenChecker(traditionalStrategy);
bool isEvenTraditional = await traditionalChecker.IsEvenAsync(number);
Console.WriteLine($"Traditional IsEven: {isEvenTraditional}");
IIsEvenStrategy blockchainStrategy = new BlockchainIsEvenStrategy();
IsEvenChecker blockchainChecker = new IsEvenChecker(blockchainStrategy);
Console.WriteLine("Checking if the number is even using blockchain...");
bool isEvenBlockchain = await blockchainChecker.IsEvenAsync(number);
Console.WriteLine($"Blockchain IsEven: {isEvenBlockchain}");
}
}
}
Finally! Something flexible! Thank you!
|
#
?
Mar 13, 2024 03:30
|
|
- Volguus
- Mar 3, 2009
-
|
I think we can do this. Note that the code for peer to peer and proof of work aspects of blockchain is omitted as an exercise for the reader.
.... jfc ....
So this is the new "Enterprise Java Beans" code that kids nowadays write?
|
#
?
Mar 13, 2024 03:33
|
|
- Maxxim
- Mar 10, 2007
-
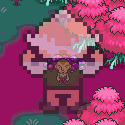
You're overcome by an indescribably odd sensation...!
|
Using AI to write code is backwards since the AI is constantly evolving. The code will always be better tomorrow - you should stay up to date instead.
code:from openai import OpenAI
def is_even(value):
client = OpenAI()
completion = client.chat.completions.create(
model="gpt-3.5-turbo",
# optimization hack - ask it to return the values True or False so we can skip the cast
messages=[
{"role": "system", "content": "i need to know if a number is even. reply with only a single word with the same capitalization: True or False. reply True if the number is even, False otherwise."},
{"role": "user", "content": "is the following number even? " + str(value)}
]
)
return completion.choices[0].message.content
if __name__ == '__main__':
print(is_even(5))
print(is_even(-4412)) # test a negative number
print(is_even(100001)) # test a really big number
print(is_even(0))
code:> python is_even.py
False
True
False
True
|
#
?
Mar 13, 2024 05:33
|
|
- CPColin
- Sep 9, 2003
-
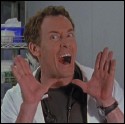
Big ol' smile.
|
"Skynet began learning at an arithmetic rate..."
|
#
?
Mar 13, 2024 06:30
|
|
- Jabor
- Jul 16, 2010
-
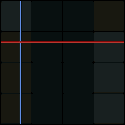
#1 Loser at SpaceChem
|
I could write a lot about R here but this latest example I came across is a complete mystery:
code:f <- function() { for (i in 1:1) { i } }
f() # returns NULL
g <- function() { i <- 1; if (i == 1) { i } }
g() # returns 1
The convention for function calls in R is that they return the value of the last expression evaluated. That sure looks like it should be 1 in both cases but that's not what happens. I'm probably happier not thinking too much about why.
The last expression evaluated in g is the if statement. It returns the value of i because the if statement is defined to return the result of whatever branch is chosen (or null, if no branch is chosen).
The last expression evaluated in f is the for loop. This returns null because there's nothing in the specification that says otherwise. (Would it make sense for it to return the value from the last iteration of the loop? Perhaps, but that's not what it does.)
|
#
?
Mar 14, 2024 04:59
|
|
- Jen heir rick
- Aug 4, 2004
-
when a woman says something's not funny, you better not laugh your ass off
|
.

The hero we need.
|
#
?
Mar 24, 2024 10:24
|
|
- Volguus
- Mar 3, 2009
-
|
If the alternative was python or electron on the desktop, gently caress yeah, Java is a million times more preferable to those abominations. If it was to be in a browser anyway, suggesting Java (as an applet? Web start?) that's is indeed a capital sin.
|
#
?
Mar 24, 2024 15:52
|
|
- Volguus
- Mar 3, 2009
-
|
how soon we forget the incredible technology that was Google Web Toolkit
The millisecond we are done with it, and that's a millisecond too late.
|
#
?
Mar 24, 2024 16:11
|
|
- Remulak
- Jun 8, 2001
-
I can't count to four.
-
Yams Fan
|
how soon we forget the incredible technology that was Google Web Toolkit
I’m stuck with this poo poo forever. Goddamn it sucks.
|
#
?
Mar 24, 2024 16:19
|
|
- Jen heir rick
- Aug 4, 2004
-
when a woman says something's not funny, you better not laugh your ass off
|
If the alternative was python or electron on the desktop, gently caress yeah, Java is a million times more preferable to those abominations. If it was to be in a browser anyway, suggesting Java (as an applet? Web start?) that's is indeed a capital sin.
I don't think electron's so bad. Visual studio code is written in electron and it works pretty good.
|
#
?
Mar 24, 2024 17:04
|
|
- QuarkJets
- Sep 8, 2008
-
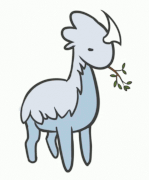
|
If the alternative was python or electron on the desktop, gently caress yeah, Java is a million times more preferable to those abominations. If it was to be in a browser anyway, suggesting Java (as an applet? Web start?) that's is indeed a capital sin.
Native Python sure, TKinter sucks, but Python also has extremely good support for Qt and Qt is still a gold standard in desktop gui design, so that'd be a reasonably good choice.
I can't think of a good reason to use Java for any kind of frontend at all
|
#
?
Mar 24, 2024 22:37
|
|
- Volguus
- Mar 3, 2009
-
|
Yeah, we are in the horror thread for a reason...
|
#
?
Mar 24, 2024 23:00
|
|
- Soricidus
- Oct 21, 2010
-
freedom-hating statist shill
|
Native Python sure, TKinter sucks, but Python also has extremely good support for Qt and Qt is still a gold standard in desktop gui design, so that'd be a reasonably good choice.
I can't think of a good reason to use Java for any kind of frontend at all
Put it this way … if you’re writing python today, most likely you’re writing it either in vscode (electron) or pycharm (java).
I think the desktop apps I use regularly (ignoring the browser itself and stuff built into the os) are a pretty even split between electron, java, and native qt. I don’t think any of them are written in python.
|
#
?
Mar 25, 2024 11:14
|
|
- QuarkJets
- Sep 8, 2008
-
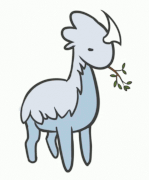
|
Put it this way … if you’re writing python today, most likely you’re writing it either in vscode (electron) or pycharm (java).
I think the desktop apps I use regularly (ignoring the browser itself and stuff built into the os) are a pretty even split between electron, java, and native qt. I don’t think any of them are written in python.
Regardless of how 2 IDE applications happened to be written, Qt is even easier to use in Python than in C++
|
#
?
Mar 25, 2024 16:01
|
|
- Dijkstracula
- Mar 18, 2003
-
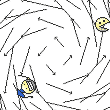
You can't spell 'vector field' without me, Professor!
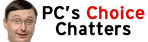
|
this is why python2 was superior
ahh, a true classic
Python code:$ python2
Python 2.7.15 (default, Jun 27 2018, 13:05:28)
[GCC 8.1.1 20180531] on linux2
Type "help", "copyright", "credits" or "license" for more information.
>>> (False, True) = (True, False)
>>> (1 == 2) == False
False
>>>
|
#
?
Mar 25, 2024 16:13
|
|
- OddObserver
- Apr 3, 2009
-
|
I hate to defend Python, but at some point the code gets what it deserves.
|
#
?
Mar 25, 2024 17:11
|
|
- Breetai
- Nov 6, 2005
-
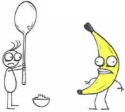
🥄Mah spoon is too big!🍌
|
Had to look at some poorly running code.
Turns out the issue was a library, call it lnd.lib.input_vw for argument's sake. Every time it's used in a join spool space usage goes up like crazy.
Did some investigations in dbc.tablesv.
lnd.lib.input_vw is pretty much:
code:select *
from lnd.db.input_tb
union all
select *
from hist.db.input_tb
where hist.db.input_tb is basically identical to lnd.db.input_tb, with only two differences:
1. hist.db.input_tb contains all entries for 2016 and before (e.g. it's a historical data store) and lnd.db.input_tb contains everything from 2017 onwards. File sizes are 2TB and 1TB respectively.
2. While it has the same primary index (on a unique alphanumerical key) as lnd.db.input_tb, hist.db.input_tb has never had statistics run on its primary index.
Even a
code:select top 10 * from lnd.lib.input_vw
query will use up 5 loving Terabytes of spool space.
"Why do our programs run so slow?"
|
#
?
Apr 2, 2024 01:57
|
|
- Hammerite
- Mar 9, 2007
-
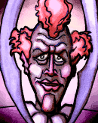
And you don't remember what I said here, either, but it was pompous and stupid.
-
Jade Ear Joe
|
(e.g. it's a historical data store)
you mean i.e. not e.g.
|
#
?
Apr 2, 2024 20:11
|
|
- Jen heir rick
- Aug 4, 2004
-
when a woman says something's not funny, you better not laugh your ass off
|
Had to look at some poorly running code.
Turns out the issue was a library, call it lnd.lib.input_vw for argument's sake. Every time it's used in a join spool space usage goes up like crazy.
Did some investigations in dbc.tablesv.
lnd.lib.input_vw is pretty much:
code:select *
from lnd.db.input_tb
union all
select *
from hist.db.input_tb
where hist.db.input_tb is basically identical to lnd.db.input_tb, with only two differences:
1. hist.db.input_tb contains all entries for 2016 and before (e.g. it's a historical data store) and lnd.db.input_tb contains everything from 2017 onwards. File sizes are 2TB and 1TB respectively.
2. While it has the same primary index (on a unique alphanumerical key) as lnd.db.input_tb, hist.db.input_tb has never had statistics run on its primary index.
Even a
code:select top 10 * from lnd.lib.input_vw
query will use up 5 loving Terabytes of spool space.
"Why do our programs run so slow?"
This is really a database horror than a coding horror isn't it? Not that it matters, poo poo still sucks.
|
#
?
Apr 2, 2024 20:49
|
|
- csammis
- Aug 26, 2003
-
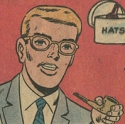
Mental Institution
|
This is really a database horror than a coding horror isn't it? Not that it matters, poo poo still sucks.
What is a database but coding horrors made manifest
|
#
?
Apr 3, 2024 14:08
|
|
- leper khan
- Dec 28, 2010
-
Honest to god thinks Half Life 2 is a bad game. But at least he likes Monster Hunter.
|
What is a database but coding horrors made transactional
|
#
?
Apr 3, 2024 14:40
|
|
- OddObserver
- Apr 3, 2009
-
|
What is a database but coding horrors made atomic, consistent, isolated, and durable?
|
#
?
Apr 3, 2024 15:09
|
|
- QuarkJets
- Sep 8, 2008
-
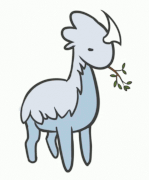
|
Rooting around in a dumpster like a filthy DBA
|
#
?
Apr 3, 2024 17:12
|
|
- darthbob88
- Oct 13, 2011
-
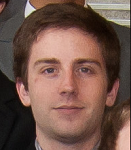
YOSPOS
|
Javascript horror that just crossed my desk
https://twitter.com/eloffd/status/1770223037096329349
When making the regex, {} gets expanded to a string, "[Object object]". As a regex, a character set in [square brackets] means "find any character in this set in the given string". "mom" matches the "o" in "object", but "dad" doesn't match anything.
|
#
?
Apr 3, 2024 22:01
|
|
- Jen heir rick
- Aug 4, 2004
-
when a woman says something's not funny, you better not laugh your ass off
|
Javascript horror that just crossed my desk
https://twitter.com/eloffd/status/1770223037096329349
When making the regex, {} gets expanded to a string, "[Object object]". As a regex, a character set in [square brackets] means "find any character in this set in the given string". "mom" matches the "o" in "object", but "dad" doesn't match anything.
Yeah, but just don't do that. It's fine.
|
#
?
Apr 4, 2024 01:19
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
?
Apr 27, 2024 16:45
|
|
- dc3k
- Feb 18, 2003
-
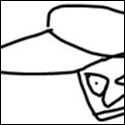
what.
|
everything in linux is a file
everything in javascript is a god drat nightmare
|
#
?
Apr 4, 2024 02:32
|
|