- Yaoi Gagarin
- Feb 20, 2014
-
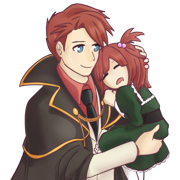
|
I'm following vulkan-tutorial.com on an old mac with integrated graphics. I ran into a weird issue, and I wonder if any of y'all have a clue about what could be going on.
The first part of the tutorial involves drawing a single triangle to the screen without using a vertex buffer at all, so the vertex shader just hardcodes the vertex positions and colors into the shader, using gl_VertexIndex to select the appropriate position/color. I'm following the code exactly to the tutorial, but my triangle's colors are wrong: only one corner is red, and the other two corners are black.
code:// Vertex shader
#version 450
layout(location = 0) out vec3 vertColor;
vec2 positions[3] = vec2[](
vec2(0.0, -0.5),
vec2(0.5, 0.5),
vec2(-0.5, 0.5)
);
vec3 colors[3] = vec3[](
vec3(1.0, 0.0, 0.0),
vec3(0.0, 1.0, 0.0),
vec3(0.0, 0.0, 1.0)
);
void main() {
gl_Position = vec4(positions[gl_VertexIndex], 0.0, 1.0);
vertColor = colors[gl_VertexIndex];
}
The fragment shader is super simple, but including it just for completeness:
code:// fragment shader
#version 450
layout(location = 0) in vec3 vertColor;
layout(location = 0) out vec4 outColor;
void main() {
outColor = vec4(vertColor, 1.0);
}
HOWEVER, here's the weird part: if I change main to re-set the values of the colors array individually, the result looks perfectly fine:
code:// <above main, all the code is the same>
void main() {
gl_Position = vec4(positions[gl_VertexIndex], 0.0, 1.0);
colors[0] = vec3(1.0, 0.0, 0.0);
colors[1] = vec3(0.0, 1.0, 0.0);
colors[2] = vec3(0.0, 0.0, 1.0);
vertColor = colors[gl_VertexIndex];
}
Does anyone have any idea why the 2nd main() function would work where the 1st one didn't? There seems to be something odd about the array of vec3's. But the weird thing is that the array of vec2's before that for the positions works just fine.
e: I realize that this specific shader is totally unrealistic, but I'm stuck on this because in the future if I want to use hardcoded constant arrays for some reason, I'm not gonna trust 'em if they can exhibit weird behavior I don't understand!!!

This could very well be a compiler bug. Can you post the SPIR-V? The Vulkan sdk has a program called spirv-dis you can use to get a text listing of a SPIR-V binary. I'm not familiar with Mac development but I assume you're using glslang to convert from GLSL to SPIR-V right?
Suppose I have a compute shader with a really sparse SSBO array that is ostensibly a tree structure that fills itself up (fixed depth, fixed size). Is it fine to just no-op any indexes that haven't been populated yet?
Edit: Found better leverage of my acceleration structure to still do everything parallel but get 90% of the benefit. Still posing the question whether a single if-then-no-op is potentially slow just because it needs to split the execution weirdly or something.
If you mean something like:
code:
if (is_populated[threadID]) {
// Do stuff
}
It'll really depend on how much work is done on that branch. If it's really quick then it's probably not a big deal but if it's long then you could be hurting your utilization over time.
Note that if you have an else branch on that it gets even worse because all the threads in each warp will have to run both if even one is different
|
#
¿
Apr 15, 2023 19:59
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
Apr 29, 2024 16:00
|
|