- Ghost of Reagan Past
- Oct 7, 2003
-
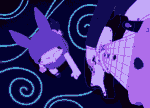
rock and roll fun
|
Here's the library: https://github.com/jdeeny/rust-chip8
And here's the SDL2 emulator application: https://github.com/jdeeny/vipchip
The emulator app is pretty bare. cargo run examples/computer.hex will run it. It will run code from a file filled with hex data like you get from Octo or a binary file. Escape to exit.
A few weeks ago I had everything working to about the same point as this, but that time it was all one monolithic app with no tests. This is the result of trying to split it into the UI and a library to handle the core chip8 simulation task. I've gone round in circles on more than a few design decisions in the library.
I used locks on the state data that I thought the UI would want to have fast access to, and channels for the other stuff. Honestly, I just wanted to try both. It sure seems like locks will be faster, but it intrudes on the code everywhere. Curious about a more ergonomic way to do this.
I started with the Operations as classes implementing a trait, but there was a ton of boilerplate so I switched to Enums. I found out about default implementations, so maybe I should switch back. I really don't like how the enums have un-named data. Especially now that I have some operations with flags, it is really ugly.
Can I do Src/SrcKind and Dest/DestKind in a better way? Maybe I need a macro.
Known problem: SimulatorTask needs a run function that will execute instructions autonomously, without the other thread stepping it. Right now it is sort of pointless.
Cool coincidence, I decided I wanted to learn some Rust this evening and I started writing a Chip-8 emulator 
I'm still trying to wrap my head around memory and all that; I'm used to Python and Haskell, and don't know any C or C++, but Rust seems neat (and this emulator project is cool; I've never done anything with systems code at all and it's very educational). I'm sure mine is a monstrosity of non-idiomatic Rust, but oh well. It reads instructions and does things, though I don't have any drawing implemented so if it's actually doing the right things.
Can someone answer my extreme noob question? This feels like it should be valid Rust
code:fn main() {
let mut x: u32 = 5;
reassign(&mut x); // type mismatch
}
fn reassign(x: &mut u32) {
x = 4;
}
Why is it not valid Rust? x is mutable, and the function borrows a mutable x, but the docs don't explain why this isn't valid as far as I can tell. I know it works if you pass a struct and change a value on the struct, with the exact same pattern (this is what the docs have as examples), but rustc keeps telling me about a type mismatch with this.
Another question: is there a way to get Cargo to update rustc? They release a new stable every 6 weeks, and so if I want to keep my local updated, how do I do that except uninstall old versions and install the new one (Windows)?
Ghost of Reagan Past fucked around with this message at 02:24 on Aug 22, 2016
|
#
¿
Aug 22, 2016 02:11
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 5, 2024 02:08
|
|
- Ghost of Reagan Past
- Oct 7, 2003
-
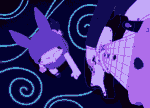
rock and roll fun
|
Oh I forgot to add the &mut when I passed the function in the example.
It was in the original code so I edited it above (this is just a toy example, I just ran against it earlier).
This is the error I get
code:src\main.rs:41:9: 41:10 note: expected type `&mut u32
src\main.rs:41:9: 41:10 note: found type `_`
My understanding is that the 'reassign' function is borrowing x, and since it's mutably borrowing it, it should be allowed to change it.
Ghost of Reagan Past fucked around with this message at 02:32 on Aug 22, 2016
|
#
¿
Aug 22, 2016 02:27
|
|
- Ghost of Reagan Past
- Oct 7, 2003
-
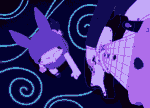
rock and roll fun
|
It was complaining that I was trying to change a reference (that much I understood). I'm borrowing it via the reference, but if I want to do something to the thing, I gotta make sure I'm talking about the thing, not the reference. This makes sense now.
|
#
¿
Aug 22, 2016 02:42
|
|