- Klades
- Sep 8, 2011
-
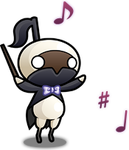
|
Which is where my brain went right away after being primed with FizzBuzz solutions. In pseudo code:
code:int input = XXX;
int count =0;
for(int i = 31; i >= 0; i--){
int powerOf2 = 2^i;
if(input % powerOf2 == 0){
count++;
input -= powerOf2;
}
}
Generally by refactoring you should not make things worse than they were when you got there. Raising to the power of i basically means you just made a nested loop. Plus, the code doesn't actually give the correct results, at least in C++. I set input to 12 and got 3, set it to 13 and got 1, set it to 2 and got 2.
If you really wanted to change the code so that someone who didn't know about bitwise operators could look at it and tell you what it was doing, it'd be something like this
code:int count_bits (int number) {
int bits = 0;
while (number) {
// Check for odd-ness
if (number % 2) {
++bits;
}
// Shift all bits one to the right
number >>= 1;
}
return bits;
}
If someone can't at least understand that maybe they aren't a good programmer.
|
#
¿
Jun 19, 2016 18:56
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 11, 2024 09:31
|
|
- Klades
- Sep 8, 2011
-
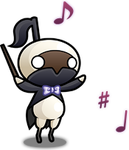
|
code:private Map<string,int> Count(string input)
{
string word = "apache";
int length = word.length;
Map<string, int> counts = new HashMap<string,int>();
for(int i = 0; i < length; i ++)
{
if(counts[i] != null)
{
counts[i]++;
}
}
return counts;
}
I wonder how, even supposing that worked, this would ever not return an empty map, since each letter-key would not exist at first, and therefore wouldn't be incremented.
Even better, if you've represented this accurately all it does is make a new HashMap, then increment the first six elements of that map if they exist, which they don't because it's a fresh, empty map.
|
#
¿
Aug 9, 2016 22:05
|
|