- SurgicalOntologist
- Jun 17, 2004
-
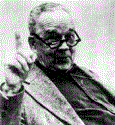
|
For issues like syntax and function signatures, the official docs are your best bet. I refer to them all the time. docs.python.org/3
|
#
?
Aug 21, 2014 06:05
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
?
May 9, 2024 00:33
|
|
- Literally Elvis
- Oct 21, 2013
-
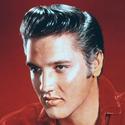
|
So I was reading about test-driven development, and I'm trying it out by implementing suuuuuuper simple algorithms in Python. Initially using everybody's favorite: bubble sort.
So at first I wrote this:
Python code:import unittest
import bubble
class SortTest(unittest.TestCase):
unsorted = [62, 55, 99, 48, 23, 88, 21, 10, 24, 49]
_sorted = [10, 21, 23, 24, 48, 49, 55, 62, 88, 99]
def test_bubble_sort(self):
'''bubble_sort should sort unsorted list'''
result = bubble.sort(self.unsorted)
self.assertEqual(result, self._sorted)
if __name__ == '__main__':
unittest.main()
Because what I read said to write tests that fail first, then code until they pass. After everything was working, though, I re-wrote the test because part of me thought that would be a better way to test the function would be to compare it against Python's own built in sort() function:
Python code:import unittest
import random
import bubble_sort as bubble
class SortTest(unittest.TestCase):
unsorted = []
for x in range(0, 20):
unsorted.append(random.randint(0, 100))
_sorted = unsorted
_sorted.sort()
def test_bubble_sort(self):
'''bubble_sort should sort unsorted list'''
result = bubble.sort_list(self.unsorted)
self.assertEqual(result, self._sorted)
if __name__ == '__main__':
unittest.main()
But now I'm wondering if it's a good idea at all to compare a function I wrote with a built-in function, even though it's relatively simple.
|
#
?
Aug 21, 2014 07:41
|
|
- Symbolic Butt
- Mar 22, 2009
-
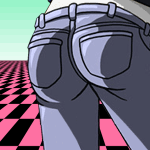
(_!_)
-
Buglord
|
IMO you should've kept both tests. The one with your hardcoded sorted data and the one testing against the built-in sort.
Imagine how interesting it'd be if only one of those tests fail.
|
#
?
Aug 21, 2014 09:46
|
|
- Literally Elvis
- Oct 21, 2013
-
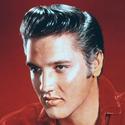
|
IMO you should've kept both tests. The one with your hardcoded sorted data and the one testing against the built-in sort.
Imagine how interesting it'd be if only one of those tests fail.
I like (and have implemented) that idea.
Is 7 tests for a loving numeric bubble sorter too many or too few?
|
#
?
Aug 21, 2014 10:06
|
|
- Jewel
- May 2, 2009
-
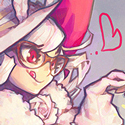
|
Also; I don't know if I'm a fan of "bubble.sort". To me it feels like it should be "sort.bubble" or similar. Because then you could have various other sort options in the same "sort" library.
|
#
?
Aug 21, 2014 10:10
|
|
- 'ST
- Jul 24, 2003
-
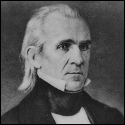
"The world has nothing to fear from military ambition in our Government."
|
But now I'm wondering if it's a good idea at all to compare a function I wrote with a built-in function, even though it's relatively simple.
I think it's a great idea if the built-in implementation gives accurate results. Python's sorting is going to be solid.
One issue:
Python code:...
unsorted = []
for x in range(0, 20):
unsorted.append(random.randint(0, 100))
_sorted = unsorted
_sorted.sort()
...
list.sort() sorts a list object in place. Since _sorted = unsorted is just copying a reference to the same list object, _sorted.sort() is equivalent to unsorted.sort() in that code.
Either explicitly copy the unsorted list before sorting or use the built-in sorted which returns a new sorted list:
Python code:...
unsorted = []
for x in range(0, 20):
unsorted.append(random.randint(0, 100))
_sorted = sorted(unsorted)
...
There are a lot of behaviors in Python where it's useful to understand how the language treats object references, but you can find those as you go along.
|
#
?
Aug 21, 2014 13:45
|
|
- OnceIWasAnOstrich
- Jul 22, 2006
-
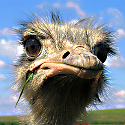
|
To be honest, I can't remember why we recommended against it in the past...
A part of the problem was the book was written for Python 2.1/2 and hasn't been updated since Python 2.3, so it was missing about seven years of Python 2.x development. A version specifically for 3.x presumably wouldn't have that problem.
|
#
?
Aug 21, 2014 16:45
|
|
- Plorkyeran
- Mar 22, 2007
-
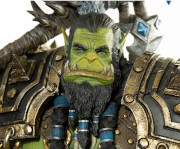
To Escape The Shackles Of The Old Forums, We Must Reject The Tribal Negativity He Endorsed
|
To be honest, I can't remember why we recommended against it in the past...
It's comically out of date and tells you to do lots of terrible things as a result, and large chunks of it are entirely irrelevant now (such as the chapters on ODBC).
|
#
?
Aug 21, 2014 16:57
|
|
- ShadowHawk
- Jun 25, 2000
-
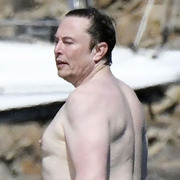
CERTIFIED PRE OWNED TESLA OWNER
|
If you're a true beginner to programming, I'm going to recommend Think Python (and start with the version adapted to be Python 3 only). It's a free PDF too.
|
#
?
Aug 21, 2014 17:27
|
|
- Literally Elvis
- Oct 21, 2013
-
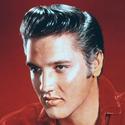
|
Also; I don't know if I'm a fan of "bubble.sort". To me it feels like it should be "sort.bubble" or similar. Because then you could have various other sort options in the same "sort" library.
Yeah, I've been thinking a lot about how I'm going to organize all of these. I sort of like the idea of having one main sort.py and things like bubble_test.py and quicksort_test.py for the testing pages, but that can all be revised later.
One issue:
list.sort() sorts a list object in place. Since _sorted = unsorted is just copying a reference to the same list object, _sorted.sort() is equivalent to unsorted.sort() in that code.
Either explicitly copy the unsorted list before sorting or use the built-in sorted which returns a new sorted list:
Python code:...
unsorted = []
for x in range(0, 20):
unsorted.append(random.randint(0, 100))
_sorted = sorted(unsorted)
...
There are a lot of behaviors in Python where it's useful to understand how the language treats object references, but you can find those as you go along.
I wasn't super stoked about how the code I wrote read, and this is much better. Thanks.
We've always recommended against dive into python in this thread, but I don't know if the version for Python 3 has improved?
To be honest, I can't remember why we recommended against it in the past...
I've been going through the 3 version for the last two weeks or so when I have time, and I feel like it's really solid. I started reading it because while I had written some scripts successfully in the past, and I could explain what everything did, I felt like there were a lot of things about the language that I didn't understand (see above for an example). Like all books, it's got its flaws. It's not for beginners, but I wasn't really new to programming when I started reading it.
|
#
?
Aug 21, 2014 18:22
|
|
- JHVH-1
- Jun 28, 2002
-
|
This might not cover everything you would need to know, but I really like how this site lays things out http://www.dotnetperls.com/python
|
#
?
Aug 21, 2014 18:28
|
|
- ShadowHawk
- Jun 25, 2000
-
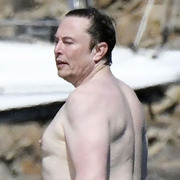
CERTIFIED PRE OWNED TESLA OWNER
|
Annotations are awesome. My only complaint is there's no particularly easy way to annotate a function as returning another function. You can annotate strings and ints using str and int and the like, but there's no function primitive (lambda won't work without being defined).
Speaking of annotations, I'm getting curious about optimizing a bit of code using cython, but it seems to require its own special annotations rather than just being smart enough to use the Python3 annotations. Has anyone had experience compiling with cython?
|
#
?
Aug 21, 2014 22:11
|
|
- accipter
- Sep 12, 2003
-
|
Annotations are awesome. My only complaint is there's no particularly easy way to annotate a function as returning another function. You can annotate strings and ints using str and int and the like, but there's no function primitive (lambda won't work without being defined).
Speaking of annotations, I'm getting curious about optimizing a bit of code using cython, but it seems to require its own special annotations rather than just being smart enough to use the Python3 annotations. Has anyone had experience compiling with cython?
Have you looked at using Numba over Cython? This comparison shows that Numba is pretty similar in speed, and much simpler to use.
|
#
?
Aug 21, 2014 22:42
|
|
- fritz
- Jul 26, 2003
-
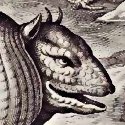
|
Is 7 tests for a loving numeric bubble sorter too many or too few?
Depends on the tests. Is everything being tested that's reasonable to test? Are they fast to run? Are they informative?
|
#
?
Aug 22, 2014 03:56
|
|
- Suspicious Dish
- Sep 24, 2011
-
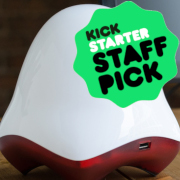
2020 is the year of linux on the desktop, bro
-
Fun Shoe
|
We've always recommended against dive into python in this thread, but I don't know if the version for Python 3 has improved?
To be honest, I can't remember why we recommended against it in the past...
It has not. Somewhere on the internet is a document we compiled of all the mistakes in DIP3, archived from a Google Wave document. I couldn't find it on my phone, but search for habnabit, papna, marienz and others.
|
#
?
Aug 22, 2014 04:42
|
|
- Literally Elvis
- Oct 21, 2013
-
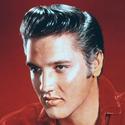
|
It has not. Somewhere on the internet is a document we compiled of all the mistakes in DIP3, archived from a Google Wave document. I couldn't find it on my phone, but search for habnabit, papna, marienz and others.
I can't find those at all, nor can I seem to find any scathing criticism from any of the book's reviewers. Like, the Amazon page for the book has negative reviews of the print quality, and there are some complaints elsewhere that the book wasn't targeted towards beginners. But I haven't found anything that says "god drat this book is trash." I'm really interested to read what those complaints were, though, so if somebody could find that discussion or reiterate their points, I'd love to see it.
|
#
?
Aug 22, 2014 05:02
|
|
- ohgodwhat
- Aug 6, 2005
-
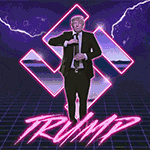
|
Have you looked at using Numba over Cython? This comparison shows that Numba is pretty similar in speed, and much simpler to use.
Someone I work with who is otherwise a very competent dude was adamant recently that numba and cython are almost identical; not in effect, but actual implementation. My understanding was that numba produces LLVM IR on the fly that gets executed in its virtual machine (or whatever), while cython is more of a static thing - producing C that is then compiled. Am I on the right track?
I'm a big fan of Numba, too.
|
#
?
Aug 23, 2014 02:39
|
|
- ShadowHawk
- Jun 25, 2000
-
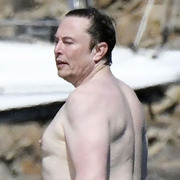
CERTIFIED PRE OWNED TESLA OWNER
|
Have you looked at using Numba over Cython? This comparison shows that Numba is pretty similar in speed, and much simpler to use.
I looked at both, then reprofiled my app, and realized that the function I was stuck in was doing a lot of membership testing within a set, and I don't think typifying either of them will help with that.
Numba, for instance, would error when I @jit that single function.
|
#
?
Aug 23, 2014 02:43
|
|
- BigRedDot
- Mar 6, 2008
-

|
Someone I work with who is otherwise a very competent dude was adamant recently that numba and cython are almost identical; not in effect, but actual implementation. My understanding was that numba produces LLVM IR on the fly that gets executed in its virtual machine (or whatever), while cython is more of a static thing - producing C that is then compiled. Am I on the right track?
That is exactly correct. To add more context, compiling python for performance is always all about obtaining type information upon which to specialize on. Cython does it with explicit annotations and separate compilation step, PyPy does it with an instrumented interpreter that performs tracing data-flow analysis, and Numba does it by using NumPy type information and optional runtime arguments to emit LLVM byte code..
|
#
?
Aug 23, 2014 03:52
|
|
- ShadowHawk
- Jun 25, 2000
-
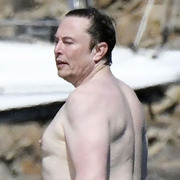
CERTIFIED PRE OWNED TESLA OWNER
|
That is exactly correct. To add more context, compiling python for performance is always all about obtaining type information upon which to specialize on. Cython does it with explicit annotations and separate compilation step, PyPy does it with an instrumented interpreter that performs tracing data-flow analysis, and Numba does it by using NumPy type information and optional runtime arguments to emit LLVM byte code..
And yet, none of them do it via Python 3's function annotations :/
Although Cython at least has that as a todo list item.
|
#
?
Aug 23, 2014 05:41
|
|
- BigRedDot
- Mar 6, 2008
-

|
And yet, none of them do it via Python 3's function annotations :/
Although Cython at least has that as a todo list item.
Backport function annotations to a python 2.8 and you'd probably have that next. week. That said, Numda devs are not unaware, but are waiting for the current discussions over MyPy style annotations to settle, since Guido has expressed apparent interest in them: https://github.com/numba/numba/issues/229
|
#
?
Aug 23, 2014 15:58
|
|
- Tacos Al Pastor
- Jun 20, 2003
-
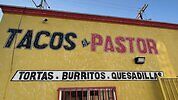
|
Got a little problem with Doctest. Im wondering why my output is throwing two lists. My code for solving the problem looks right because the second list
that it "got". Im thinking it has to do with my local variables and function instantiation issues(?). Not really sure. If Doctest got what it expected I shouldnt see anything in the output, not the output below. Any hints??
Bolded the part where the two lists are shown below.
quote:
**********************************************************************
File "/Users/spiralbrain/Google Drive/Python/Refactoring_Final_Project.py", line 9, in Refactoring_Final_Project.book_title
Failed example:
book_title('DIVE Into python')
Expected:
'Dive into Python'
Got:
['dive', 'into', 'python']
['Dive', 'into', 'Python']
**********************************************************************
File "/Users/spiralbrain/Google Drive/Python/Refactoring_Final_Project.py", line 12, in Refactoring_Final_Project.book_title
Failed example:
book_title('the great gatsby')
Expected:
'The Great Gatsby'
Got:
['the', 'great', 'gatsby']
['The', 'Great', 'Gatsby']
**********************************************************************
File "/Users/spiralbrain/Google Drive/Python/Refactoring_Final_Project.py", line 15, in Refactoring_Final_Project.book_title
Failed example:
book_title('the WORKS OF AleXANDer dumas')
Expected:
'The Works of Alexander Dumas'
Got:
['the', 'works', 'of', 'alexander', 'dumas']
['The', 'Works', 'of', 'Alexander', 'Dumas']
**********************************************************************
1 items had failures:
3 of 3 in Refactoring_Final_Project.book_title
***Test Failed*** 3 failures.
Heres my code:
Python code:small_words = ('into', 'the', 'a', 'of', 'at', 'in', 'for', 'on')
def book_title(title):
""" Takes a string and returns a title-case string.
All words EXCEPT for small words are made title case
unless the string starts with a preposition, in which
case the word is correctly capitalized.
>>> book_title('DIVE Into python')
'Dive into Python'
>>> book_title('the great gatsby')
'The Great Gatsby'
>>> book_title('the WORKS OF AleXANDer dumas')
'The Works of Alexander Dumas'
"""
newtitle = []
temp = []
count= 0
length = 0
Hlist = title.lower().split()
print(Hlist)
length = len(Hlist)
for item in Hlist:
if item in small_words and count < length:
if count == 0:
temp = Hlist[count].title()
newtitle.append(temp)
count += 1
else:
temp = Hlist[count]
newtitle.append(temp)
count += 1
elif item not in small_words and count < length:
temp = Hlist[count].title()
newtitle.append(temp)
count+=1
else:
break
return newtitle
if __name__ == "__main__":
import doctest, Refactoring_Final_Project
doctest.testmod(Refactoring_Final_Project)
edit: Holy poo poo Im an idiot. I think I got it. I printed Hlist out in the first few lines along with the output needing to be a string and not a list.
Tacos Al Pastor fucked around with this message at 23:18 on Aug 23, 2014
|
#
?
Aug 23, 2014 23:07
|
|
- ShadowHawk
- Jun 25, 2000
-
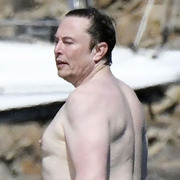
CERTIFIED PRE OWNED TESLA OWNER
|
Got a little problem with Doctest. Im wondering why my output is throwing two lists. My code for solving the problem looks right because the second list
that it "got". Im thinking it has to do with my local variables and function instantiation issues(?). Not really sure. If Doctest got what it expected I shouldnt see anything in the output, not the output below. Any hints??
Bolded the part where the two lists are shown below.
Heres my code:
Python code:small_words = ('into', 'the', 'a', 'of', 'at', 'in', 'for', 'on')
def book_title(title):
""" Takes a string and returns a title-case string.
All words EXCEPT for small words are made title case
unless the string starts with a preposition, in which
case the word is correctly capitalized.
>>> book_title('DIVE Into python')
'Dive into Python'
>>> book_title('the great gatsby')
'The Great Gatsby'
>>> book_title('the WORKS OF AleXANDer dumas')
'The Works of Alexander Dumas'
"""
newtitle = []
temp = []
count= 0
length = 0
Hlist = title.lower().split()
print(Hlist)
length = len(Hlist)
for item in Hlist:
if item in small_words and count < length:
if count == 0:
temp = Hlist[count].title()
newtitle.append(temp)
count += 1
else:
temp = Hlist[count]
newtitle.append(temp)
count += 1
elif item not in small_words and count < length:
temp = Hlist[count].title()
newtitle.append(temp)
count+=1
else:
break
return newtitle
if __name__ == "__main__":
import doctest, Refactoring_Final_Project
doctest.testmod(Refactoring_Final_Project)
edit: Holy poo poo Im an idiot. I think I got it. I printed Hlist out in the first few lines along with the output needing to be a string and not a list.
I found it faster to rewrite what you were trying to do rather than parse your code.
Python code:small_words = ('into', 'the', 'a', 'of', 'at', 'in', 'for', 'on')
def book_title(title):
""" Takes a string and returns a title-case string.
All words EXCEPT for small words are made title case
unless the string starts with a preposition, in which
case the word is correctly capitalized.
>>> book_title('DIVE Into python')
'Dive into Python'
>>> book_title('the great gatsby')
'The Great Gatsby'
>>> book_title('the WORKS OF AleXANDer dumas')
'The Works of Alexander Dumas'
"""
lower_title = title.lower().split()
out = []
def capitalize(word):
if len(word) > 1:
return word[0].upper() + word[1:]
else:
return word.upper()
for position, word in enumerate(lower_title):
if position == 0 or word not in small_words:
out.append(capitalize(word))
else:
out.append(word)
return ' '.join(out)
So, good documentation, bad code 
Python code: length = len(Hlist)
for item in Hlist:
This is a definite code smell. You almost always want to use enumerate here. The same thing goes with having a separate counter in your for loops -- it's often just unneeded in python since you can iterate over an iterator directly.
edit:
Python code:
def capitalize(word):
if len(word) > 1:
return word[0].upper() + word[1:]
else:
return word.upper()
can be simplified to:
Python code: def capitalize(word):
return word[0].upper() + word[1:]
since Python is smart enough to do the right thing with indexing a string out of bounds (returns emptystring)
ShadowHawk fucked around with this message at 23:35 on Aug 23, 2014
|
#
?
Aug 23, 2014 23:29
|
|
- dirtycajun
- Aug 27, 2004
-
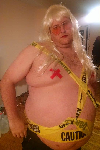
SUCKING DICKS AND SQUEEZING TITTIES
|
Hey, this is my first program ever, besides the paired arduino program to go with this one. For some reason I can't get the break in the loop to work. What am I missing here?
code:__author__ = 'DirtyCajun'
#gets stock change compared to day's open and makes an arduino light up
#(green is positive, red is negative, blue is no change)
#Only works during market hours, does not account for holidays
from yahoo_finance import Share
import serial
import time
ser = serial.Serial("COM4", 9600)
hour = float(time.strftime("%H%M", time.localtime()))
day = float(time.strftime("%w", time.localtime()))
x = 1
while True:
while hour >= 930 and hour < 1630 and day >= 1 and day <=5:
anadarko = Share('APC')
print anadarko.get_change()
if float(anadarko.get_change()) > 0:
ser.write(chr(1))
print (1)
elif float(anadarko.get_change()) == 0:
ser.write(chr(0))
else:
ser.write(chr(2))
print (2)
time.sleep(5)
if hour == 1630:
break
else:
ser.write(chr(0))
print "NYSE Closed"
time.sleep(1)
x += 1
(also any help making my code look prettier or cleaning it all up in general would be nice)
dirtycajun fucked around with this message at 03:45 on Aug 24, 2014
|
#
?
Aug 24, 2014 03:43
|
|
- Dominoes
- Sep 20, 2007
-
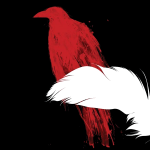
|
You're not incrementing hour. Put the time calls in the loop to fix.
|
#
?
Aug 24, 2014 03:46
|
|
- dirtycajun
- Aug 27, 2004
-
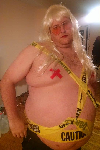
SUCKING DICKS AND SQUEEZING TITTIES
|
You're not incrementing hour. Put the time calls in the loop to fix.
I really am new to this, can you explain what you mean by that?
|
#
?
Aug 24, 2014 03:50
|
|
- Dominoes
- Sep 20, 2007
-
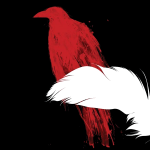
|
Python code:x = 1
while hour >= 930 and hour < 1630 and day >= 1 and day <=5:
hour = float(time.strftime("%H%M", time.localtime()))
day = float(time.strftime("%w", time.localtime()))
anadarko = Share('APC')
print anadarko.get_change()
if float(anadarko.get_change()) > 0:
ser.write(chr(1))
print (1)
elif float(anadarko.get_change()) == 0:
ser.write(chr(0))
else:
ser.write(chr(2))
print (2)
time.sleep(5)
ser.write(chr(0))
print "NYSE Closed"
time.sleep(1)
x += 1
You were pulling the current time once before entering the loop, then never updating it after.
Here's some similar, more robust code I use. It handles timezones, and market holidays. You could also use a broker's API call to ask the current market state.
Python code:def now():
return arrow.utcnow()
def market_open(date_time: arrow.Arrow, date_only=False):
"""Determine if the stock market is open."""
# Note: There's a TK API call that explicitly returns current market state.
# Does not take into account early market closes, ie half-day holidays.
with open(os.path.join(DIR_RES, 'market_holidays.txt'), 'r') as f:
holidays = [line.replace('\n', '') for line in f]
weekday = True if date_time.weekday() < 5 else False
not_holiday = True if date_time.format('YYYY-MM-DD') not in holidays else False
if date_only:
passed_time = True
else:
market_hours = market_hours_utc(st.MARKET_OPEN, st.MARKET_CLOSE)
passed_time = True if market_hours[0] <= date_time.time() <= market_hours[1] else False
if weekday and not_holiday and passed_time:
return True
return False
def market_hours_utc(open_est, close_est) -> (dt.time, dt.time):
"""Return the UTC times when the market opens and closes, based on their
EST origins."""
est = dateutil.tz.gettz('US/Eastern')
# Today's date, with EST market hours and timezone.
open_ = now().replace(hour=open_est[0], minute=open_est[1], second=0, microsecond=0, tzinfo=est)
close = open_.replace(hour=close_est[0], minute=close_est[1])
open_ = open_.to('UTC').time()
close = close.to('UTC').time()
return open_, close
if market_open(now()):
#Do stuff...
market_holidays.txt might look like this:
code:2017-01-02
2017-01-16
2017-02-20
2017-04-14
...
Dominoes fucked around with this message at 07:29 on Aug 24, 2014
|
#
?
Aug 24, 2014 03:54
|
|
- KICK BAMA KICK
- Mar 2, 2009
-
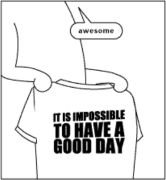
|
In addition to the above...
If this Python code:while hour >= 930 and hour < 1630 and day >= 1 and day <=5:
is the condition in the outer loop, won't Python code:if hour == 1630:
never be true? I think the break is unnecessary. Once hour == 1630 the loop will naturally break because its precondition is no longer true.
|
#
?
Aug 24, 2014 03:58
|
|
- dirtycajun
- Aug 27, 2004
-
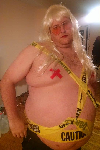
SUCKING DICKS AND SQUEEZING TITTIES
|
It won't just run forever without telling it something to stop?
Thanks for the help by the way, your explanation makes sense!
|
#
?
Aug 24, 2014 04:00
|
|
- Dominoes
- Sep 20, 2007
-
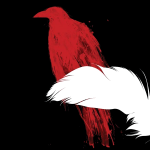
|
The while condition is what stops the loop.
|
#
?
Aug 24, 2014 04:08
|
|
- dirtycajun
- Aug 27, 2004
-
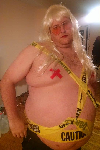
SUCKING DICKS AND SQUEEZING TITTIES
|
Okay I made the changes, turns out I did need the break, and it would only work if I did another time call in the inner loop. It can probably get cleaned up some but this functions:
code:__author__ = 'DirtyCajun'
#gets stock change compared to day's open and makes an arduino light up
#(green is positive, red is negative, blue is no change)
#Only works during market hours, does not account for holidays
from yahoo_finance import Share
import serial
import time
ser = serial.Serial("COM4", 9600)
x = 1
while True:
hour = float(time.strftime("%H%M", time.localtime()))
day = float(time.strftime("%w", time.localtime()))
while hour >= 930 and hour < 1630 and day >= 1 and day <= 5:
anadarko = Share('APC')
print anadarko.get_change()
if float(anadarko.get_change()) > 0:
ser.write(chr(1))
print (1)
elif float(anadarko.get_change()) == 0:
ser.write(chr(0))
else:
ser.write(chr(2))
print (2)
time.sleep(5)
hour = float(time.strftime("%H%M", time.localtime()))
if hour == 1630:
break
else:
ser.write(chr(0))
print "NYSE Closed"
time.sleep(1)
x += 1
It wasn't turning off just from the while function, was I doing something wrong further or will it work if I just do a time call in the inner loop to keep it updated?
edit: yea, just needed the other time call, no break. DERP
dirtycajun fucked around with this message at 04:13 on Aug 24, 2014
|
#
?
Aug 24, 2014 04:09
|
|
- Dominoes
- Sep 20, 2007
-
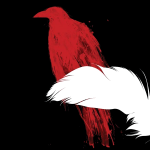
|
Get rid of the outside 'While True' loop, and set the hour inside the 'while hour' loop.
Btw, US trading hours generally end at 1600 L, not 1630.
Dominoes fucked around with this message at 05:10 on Aug 24, 2014
|
#
?
Aug 24, 2014 04:14
|
|
- dirtycajun
- Aug 27, 2004
-
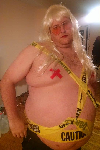
SUCKING DICKS AND SQUEEZING TITTIES
|
Thanks for the help!
I'll probably have more questions once I am trying to make this project work over a wireless network and when I am trying to make the python program figure out for itself which COM the arduino is connected to instead of being told.
|
#
?
Aug 24, 2014 04:18
|
|
- Tacos Al Pastor
- Jun 20, 2003
-
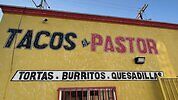
|
I found it faster to rewrite what you were trying to do rather than parse your code.
Python code:small_words = ('into', 'the', 'a', 'of', 'at', 'in', 'for', 'on')
def book_title(title):
""" Takes a string and returns a title-case string.
All words EXCEPT for small words are made title case
unless the string starts with a preposition, in which
case the word is correctly capitalized.
>>> book_title('DIVE Into python')
'Dive into Python'
>>> book_title('the great gatsby')
'The Great Gatsby'
>>> book_title('the WORKS OF AleXANDer dumas')
'The Works of Alexander Dumas'
"""
lower_title = title.lower().split()
out = []
def capitalize(word):
if len(word) > 1:
return word[0].upper() + word[1:]
else:
return word.upper()
for position, word in enumerate(lower_title):
if position == 0 or word not in small_words:
out.append(capitalize(word))
else:
out.append(word)
return ' '.join(out)
So, good documentation, bad code 
Python code: length = len(Hlist)
for item in Hlist:
This is a definite code smell. You almost always want to use enumerate here. The same thing goes with having a separate counter in your for loops -- it's often just unneeded in python since you can iterate over an iterator directly.
edit:
Python code:
def capitalize(word):
if len(word) > 1:
return word[0].upper() + word[1:]
else:
return word.upper()
can be simplified to:
Python code: def capitalize(word):
return word[0].upper() + word[1:]
since Python is smart enough to do the right thing with indexing a string out of bounds (returns emptystring)
Im still learning, so a big thanks for the pointers.
The whole point of this was to refactor some code that had some smell to it that was even worse than what I wrote, so any more improvements are appreciated.
This was the first time that I used doctest, which I get the point of but honestly I cant wait to move on to unittest. There is something about this doctest that I dont like.
|
#
?
Aug 24, 2014 05:38
|
|
- KernelSlanders
- May 27, 2013
-
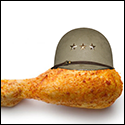
Rogue operating systems on occasion spread lies and rumors about me.
|
Okay I made the changes, turns out I did need the break, and it would only work if I did another time call in the inner loop. It can probably get cleaned up some but this functions:
...
It wasn't turning off just from the while function, was I doing something wrong further or will it work if I just do a time call in the inner loop to keep it updated?
edit: yea, just needed the other time call, no break. DERP
If your plan is to leave it running until you CTRL-C the process from the terminal, you should probably look into trapping signals so that you can shut the light off right before your application quits.
|
#
?
Aug 24, 2014 14:59
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
?
May 9, 2024 00:33
|
|
- supercrooky
- Sep 12, 2006
-
|
Anyone have any suggestions for a memory efficient 2D array of ints with reasonable indexing speed? I've gone down the list of lists route (too much memory usage) and the numpy array route (slow indexing). My next thought is wrapping the builtin array object to have a 2D like interface.
|
#
?
Aug 25, 2014 19:47
|
|